编写一段包含qt 线程的程序
时间: 2023-10-13 22:04:57 浏览: 120
好的,以下是一个简单的包含Qt线程的程序:
```
#include <QCoreApplication>
#include <QThread>
#include <QDebug>
class MyThread : public QThread {
protected:
void run() {
qDebug() << "Starting thread...";
for (int i = 0; i < 10; i++) {
qDebug() << "Thread running: " << i;
msleep(1000);
}
qDebug() << "Thread finished.";
}
};
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
MyThread thread;
thread.start();
return a.exec();
}
```
这个程序定义了一个MyThread类,继承自Qt的QThread类。在MyThread类中,我们实现了run()函数,在函数中执行了一个简单的for循环,使线程在10秒内运行。在主函数中,我们定义了一个MyThread类的实例并启动它。
相关问题
编写一段包含QT线程的程序
可以的,关于QT线程的程序,我可以帮您解答。请注意,以下代码仅供参考。
```c++
#include <QThread>
#include <QDebug>
class MyThread : public QThread {
public:
void run() override {
qDebug() << "Thread is running...";
sleep(5);
}
};
int main(int argc, char *argv[]) {
QCoreApplication a(argc, argv);
MyThread thread;
thread.start();
qDebug() << "Main thread is running...";
thread.wait();
qDebug() << "Thread is finished.";
return a.exec();
}
```
以上代码创建了一个继承自QThread的自定义类MyThread,并在其中实现了run()函数,该函数包含需要在新线程中执行的代码。
在main函数中,先创建了一个MyThread对象thread,然后调用了start()函数来启动该线程,并在新线程中执行MyThread类中的run()函数。同时,主线程继续执行。最后,主线程等待子线程执行完毕,再输出一条“Thread is finished.”的日志。
请注意,以上代码仅仅是一个简单的示例。QT线程的使用还有很多细节和需要注意的地方,具体可以参考QT官方文档。
编写一段Qt6 多线程接收串口代码
下面是一段示例 Qt6 多线程接收串口代码:#include <QThread>
#include <QDebug>
#include <QtSerialPort/QSerialPort>
#include <QtSerialPort/QSerialPortInfo>class SerialThread : public QThread
{
Q_OBJECT
public:
SerialThread(QObject *parent = nullptr);
~SerialThread();
void run();signals:
void dataReady(const QByteArray &data);private:
QSerialPort *serial;
};SerialThread::SerialThread(QObject *parent)
: QThread(parent)
{
serial = new QSerialPort(this);
connect(serial, &QSerialPort::readyRead, this, &SerialThread::run);
if (serial->open(QIODevice::ReadWrite)) {
qDebug() << "Serial port opened successfully";
} else {
qDebug() << "Serial port open failed";
}
}SerialThread::~SerialThread()
{
if (serial->isOpen())
serial->close();
}void SerialThread::run()
{
QByteArray data = serial->readAll();
emit dataReady(data);
}答案:这段代码实现了一个Qt6多线程接收串口的功能,它创建了一个SerialThread类用于读取串口,并在接收到数据时发出dataReady()信号。
阅读全文
相关推荐
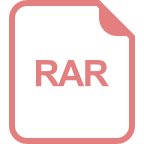
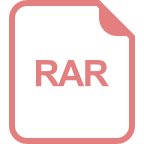
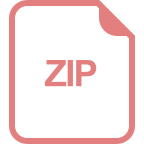













