c++语言编程实现以下排序算法: 1. 直接插入排序算法; 2. 冒泡排序算法; 3. 快速排序算法; 4. 简单选择排序算法; (要求:设置哨兵监视,使用typedef定义关键字类型)
时间: 2023-11-18 18:47:32 浏览: 88
好的,以下是对应的代码实现:
```c++
#include <iostream>
#include <ctime> //用于生成随机数
#include <cstdlib> //用于生成随机数
using namespace std;
typedef int KeyType; //关键字类型
//直接插入排序
void InsertSort(KeyType a[], int n) {
int i, j;
KeyType temp;
for (i = 1; i < n; i++) {
temp = a[i];
j = i - 1;
while (j >= 0 && temp < a[j]) {
a[j + 1] = a[j];
j--;
}
a[j + 1] = temp;
}
}
//冒泡排序
void BubbleSort(KeyType a[], int n) {
int i, j;
KeyType temp;
bool flag;
for (i = 0; i < n - 1; i++) {
flag = false;
for (j = 0; j < n - i - 1; j++) {
if (a[j] > a[j + 1]) {
temp = a[j];
a[j] = a[j + 1];
a[j + 1] = temp;
flag = true;
}
}
if (flag == false) break;
}
}
//快速排序
int Partition(KeyType a[], int low, int high) {
KeyType pivot = a[low];
while (low < high) {
while (low < high && a[high] >= pivot) high--;
a[low] = a[high];
while (low < high && a[low] <= pivot) low++;
a[high] = a[low];
}
a[low] = pivot;
return low;
}
void QuickSort(KeyType a[], int low, int high) {
if (low < high) {
int pivotPos = Partition(a, low, high);
QuickSort(a, low, pivotPos - 1);
QuickSort(a, pivotPos + 1, high);
}
}
//简单选择排序
void SelectSort(KeyType a[], int n) {
int i, j, minIndex;
KeyType temp;
for (i = 0; i < n - 1; i++) {
minIndex = i;
for (j = i + 1; j < n; j++) {
if (a[j] < a[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
temp = a[i];
a[i] = a[minIndex];
a[minIndex] = temp;
}
}
}
//生成随机数
void GenerateRandomNumbers(KeyType a[], int n) {
srand((unsigned int)time(NULL));
for (int i = 0; i < n; i++) {
a[i] = rand() % 100;
}
}
//输出数组
void PrintArray(KeyType a[], int n) {
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
}
int main() {
const int n = 10;
KeyType a[n];
GenerateRandomNumbers(a, n);
cout << "排序前:" << endl;
PrintArray(a, n);
//直接插入排序
InsertSort(a, n);
cout << "直接插入排序后:" << endl;
PrintArray(a, n);
GenerateRandomNumbers(a, n);
//冒泡排序
BubbleSort(a, n);
cout << "冒泡排序后:" << endl;
PrintArray(a, n);
GenerateRandomNumbers(a, n);
//快速排序
QuickSort(a, 0, n - 1);
cout << "快速排序后:" << endl;
PrintArray(a, n);
GenerateRandomNumbers(a, n);
//简单选择排序
SelectSort(a, n);
cout << "简单选择排序后:" << endl;
PrintArray(a, n);
return 0;
}
```
注意:为了使代码更具通用性,我在代码中添加了随机数生成函数和数组输出函数。在实际使用时,可以将这些代码注释掉。
阅读全文
相关推荐
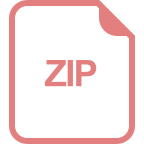
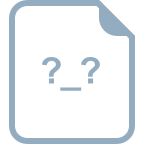

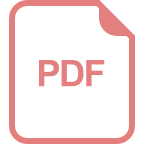
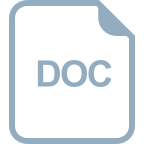
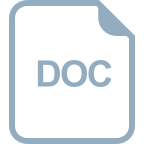
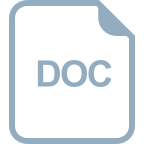
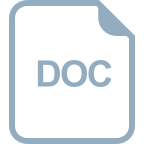
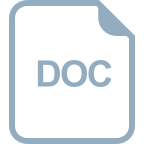
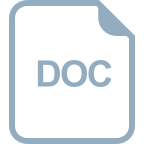
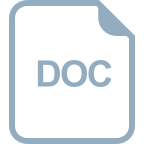
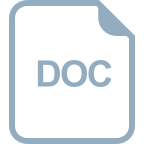
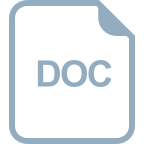
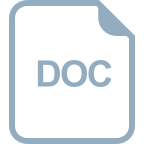
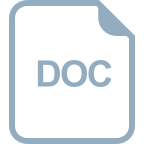

