写一个有cbam机制的网络模型
时间: 2024-06-08 10:11:38 浏览: 13
好的,我来给你写一个有CBAM机制的网络模型。CBAM是一种注意力机制,可以在卷积神经网络中加入空间和通道注意力,提升网络的性能。
这里我将以ResNet50为基础网络,加入CBAM模块,构建一个新的网络模型。
首先,我们需要导入必要的库和模块:
```python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv2D, BatchNormalization, Activation, Add, MaxPooling2D, GlobalAveragePooling2D, Dense, Multiply, Reshape
from tensorflow.keras.models import Model
```
然后,我们定义CBAM模块的两个子模块:通道注意力和空间注意力。
通道注意力模块:
```python
def channel_attention(input_feature, ratio=8):
channel_axis = 1 if tf.keras.backend.image_data_format() == "channels_first" else -1
channel = input_feature.shape[channel_axis]
shared_layer_one = Dense(channel // ratio, activation='relu', kernel_initializer='he_normal', use_bias=True, bias_initializer='zeros')
shared_layer_two = Dense(channel, kernel_initializer='he_normal', use_bias=True, bias_initializer='zeros')
avg_pool = GlobalAveragePooling2D()(input_feature)
avg_pool = Reshape((1,1,channel))(avg_pool)
avg_pool = shared_layer_one(avg_pool)
avg_pool = shared_layer_two(avg_pool)
max_pool = GlobalMaxPooling2D()(input_feature)
max_pool = Reshape((1,1,channel))(max_pool)
max_pool = shared_layer_one(max_pool)
max_pool = shared_layer_two(max_pool)
cbam_feature = Add()([avg_pool,max_pool])
cbam_feature = Activation('sigmoid')(cbam_feature)
if tf.keras.backend.image_data_format() == "channels_first":
cbam_feature = Permute((3, 1, 2))(cbam_feature)
return Multiply()([input_feature, cbam_feature])
```
空间注意力模块:
```python
def spatial_attention(input_feature, kernel_size=7):
if tf.keras.backend.image_data_format() == "channels_first":
channel = input_feature.shape[1]
cbam_feature = Permute((2,3,1))(input_feature)
else:
channel = input_feature.shape[-1]
cbam_feature = input_feature
avg_pool = Lambda(lambda x: tf.keras.backend.mean(x, axis=3, keepdims=True))(cbam_feature)
max_pool = Lambda(lambda x: tf.keras.backend.max(x, axis=3, keepdims=True))(cbam_feature)
concat = Concatenate(axis=3)([avg_pool, max_pool])
cbam_feature = Conv2D(filters = 1,
kernel_size = kernel_size,
strides=1,
padding='same',
activation='sigmoid',
kernel_initializer='he_normal',
use_bias=False)(concat)
if tf.keras.backend.image_data_format() == "channels_first":
cbam_feature = Permute((3, 1, 2))(cbam_feature)
return Multiply()([input_feature, cbam_feature])
```
接下来就是构建带有CBAM机制的ResNet50网络模型:
```python
def resnet50_cbam(input_shape, num_classes):
input_tensor = Input(shape=input_shape)
x = Conv2D(64, (7, 7), strides=(2, 2), padding='same', kernel_initializer='he_normal', name='conv1')(input_tensor)
x = BatchNormalization(name='bn_conv1')(x)
x = Activation('relu')(x)
x = MaxPooling2D((3, 3), strides=(2, 2), padding='same')(x)
x = conv_block_cbam(x, 3, [64, 64, 256], stage=2, block='a', strides=(1, 1))
x = identity_block_cbam(x, 3, [64, 64, 256], stage=2, block='b')
x = identity_block_cbam(x, 3, [64, 64, 256], stage=2, block='c')
x = conv_block_cbam(x, 3, [128, 128, 512], stage=3, block='a')
x = identity_block_cbam(x, 3, [128, 128, 512], stage=3, block='b')
x = identity_block_cbam(x, 3, [128, 128, 512], stage=3, block='c')
x = identity_block_cbam(x, 3, [128, 128, 512], stage=3, block='d')
x = conv_block_cbam(x, 3, [256, 256, 1024], stage=4, block='a')
x = identity_block_cbam(x, 3, [256, 256, 1024], stage=4, block='b')
x = identity_block_cbam(x, 3, [256, 256, 1024], stage=4, block='c')
x = identity_block_cbam(x, 3, [256, 256, 1024], stage=4, block='d')
x = identity_block_cbam(x, 3, [256, 256, 1024], stage=4, block='e')
x = identity_block_cbam(x, 3, [256, 256, 1024], stage=4, block='f')
x = conv_block_cbam(x, 3, [512, 512, 2048], stage=5, block='a')
x = identity_block_cbam(x, 3, [512, 512, 2048], stage=5, block='b')
x = identity_block_cbam(x, 3, [512, 512, 2048], stage=5, block='c')
x = GlobalAveragePooling2D(name='avg_pool')(x)
x = Dense(num_classes, activation='softmax', name='fc1000')(x)
model = Model(inputs=input_tensor, outputs=x, name='resnet50_cbam')
return model
def identity_block_cbam(input_tensor, kernel_size, filters, stage, block):
filters1, filters2, filters3 = filters
bn_axis = 3 if tf.keras.backend.image_data_format() == 'channels_last' else 1
conv_name_base = 'res' + str(stage) + block + '_branch'
bn_name_base = 'bn' + str(stage) + block + '_branch'
x = Conv2D(filters1, (1, 1), name=conv_name_base + '2a', kernel_initializer='he_normal')(input_tensor)
x = BatchNormalization(axis=bn_axis, name=bn_name_base + '2a')(x)
x = Activation('relu')(x)
x = Conv2D(filters2, kernel_size, padding='same', name=conv_name_base + '2b', kernel_initializer='he_normal')(x)
x = BatchNormalization(axis=bn_axis, name=bn_name_base + '2b')(x)
x = Activation('relu')(x)
x = Conv2D(filters3, (1, 1), name=conv_name_base + '2c', kernel_initializer='he_normal')(x)
x = BatchNormalization(axis=bn_axis, name=bn_name_base + '2c')(x)
x = Add()([x, input_tensor])
x = Activation('relu')(x)
x = channel_attention(x)
x = spatial_attention(x)
return x
def conv_block_cbam(input_tensor, kernel_size, filters, stage, block, strides=(2, 2)):
filters1, filters2, filters3 = filters
bn_axis = 3 if tf.keras.backend.image_data_format() == 'channels_last' else 1
conv_name_base = 'res' + str(stage) + block + '_branch'
bn_name_base = 'bn' + str(stage) + block + '_branch'
x = Conv2D(filters1, (1, 1), strides=strides, name=conv_name_base + '2a', kernel_initializer='he_normal')(input_tensor)
x = BatchNormalization(axis=bn_axis, name=bn_name_base + '2a')(x)
x = Activation('relu')(x)
x = Conv2D(filters2, kernel_size, padding='same', name=conv_name_base + '2b', kernel_initializer='he_normal')(x)
x = BatchNormalization(axis=bn_axis, name=bn_name_base + '2b')(x)
x = Activation('relu')(x)
x = Conv2D(filters3, (1, 1), name=conv_name_base + '2c', kernel_initializer='he_normal')(x)
x = BatchNormalization(axis=bn_axis, name=bn_name_base + '2c')(x)
shortcut = Conv2D(filters3, (1, 1), strides=strides, name=conv_name_base + '1', kernel_initializer='he_normal')(input_tensor)
shortcut = BatchNormalization(axis=bn_axis, name=bn_name_base + '1')(shortcut)
x = Add()([x, shortcut])
x = Activation('relu')(x)
x = channel_attention(x)
x = spatial_attention(x)
return x
```
这就是一个带有CBAM机制的ResNet50网络模型,可以用来进行图像分类任务。
相关推荐
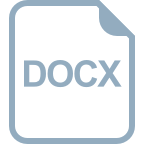
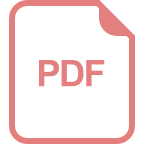















