用c语言写一段完整的代码 用插入法建立带头结点的单循环链表,实现单循环链表的初始化、求数据元素个数、插入、删除、取数据元素等操作,最后把单链表以头文件的形式保存
时间: 2024-10-08 08:13:03 浏览: 10
在C语言中,我们可以创建一个简单的单循环链表结构,并编写相应的函数实现所需操作。首先,我们需要定义链表节点和链表结构。这里是一个基本的实现:
```c
// 链表节点定义
typedef struct Node {
int data;
struct Node* next;
} Node;
// 单循环链表定义
typedef struct CircularLinkedList {
Node* head;
int size; // 链表大小
} CircularLinkedList;
// 初始化链表
void initialize(CircularLinkedList* list) {
list->head = NULL;
list->size = 0;
}
// 求链表元素个数
int get_size(CircularLinkedList* list) {
return list->size;
}
// 插入元素(在指定位置)
void insert(CircularLinkedList* list, int value, int position) {
if (position == 0 && list->head == NULL) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = newNode; // 循环链表特点:最后一个节点指向第一个
list->head = newNode;
list->size++;
return;
}
Node* prev = list->head;
for (int i = 0; i < position - 1 && prev != NULL; ++i) {
prev = prev->next;
}
if (prev != NULL) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = prev->next;
prev->next = newNode; // 插入节点
if (newNode->next == list->head) {
newNode->next = list->head; // 回到头节点
}
list->size++;
} else {
printf("Invalid position.\n");
}
}
// 删除元素(在指定位置)
void delete(CircularLinkedList* list, int position) {
if (position == 0 && list->head == NULL) {
printf("List is empty or invalid position.\n");
return;
}
Node* prev = list->head;
for (int i = 0; i < position - 1 && prev != NULL; ++i) {
prev = prev->next;
}
if (prev != NULL) {
prev->next = prev->next->next;
if (prev->next == list->head) {
list->head = list->head->next; // 走下一个节点作为新的头节点
}
free(prev->next); // 释放已删除节点的内存
list->size--;
} else {
printf("Invalid position.\n");
}
}
// 取数据元素
int get_data(CircularLinkedList* list, int index) {
if (index >= 0 && index < list->size) {
Node* current = list->head;
for (int i = 0; i <= index; i++) {
current = current->next;
}
return current->data;
} else {
printf("Invalid index.\n");
return -1;
}
}
```
请注意,这个代码示例并没有提供头文件的形式,实际应用中你需要将上述函数放入单独的.c文件中,并通过预处理器宏或者包含语句(#include "your_file.h")在需要的地方引用。同时,为了保持代码清晰,建议把这些功能封装在一个自定义的`CircularLinkedList.h`头文件中。
相关推荐
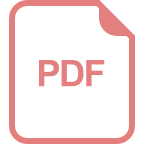
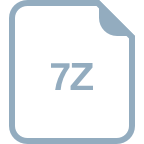
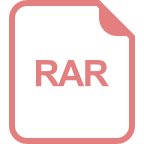











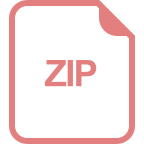
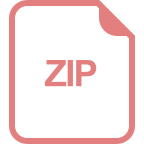