无头结点单循环链表实现约瑟夫,用C语言写出代码
时间: 2024-10-10 09:13:10 浏览: 50
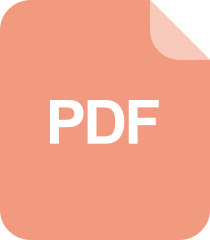
C语言单循环链表的表示与实现实例详解
在C语言中,可以使用无头节点的单循环链表来实现约瑟夫环问题,这是一个经典的算法问题,也称为“找下一个幸运数”。给定一个数组和一个整数k,你需要找出当从数组的第一个元素开始按照顺时针方向每次跳过k个元素取下一个数字,并最终返回数组中的第一个元素时,这个过程会经过多少次这样的跳跃。
下面是一个简单的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
void insertNode(Node** headRef, int data) {
Node* newNode = createNode(data);
if (*headRef == NULL) {
*headRef = newNode;
newNode->next = *headRef; // 设置单循环链接
} else {
Node* last = *headRef;
while (last->next != *headRef) {
last = last->next;
}
last->next = newNode;
newNode->next = *headRef; // 单循环链接
}
}
int josephus(Node* head, int k) {
if (head == NULL || k <= 0) {
printf("Invalid input.\n");
return -1;
}
int position = 1;
Node* current = head;
while (1) {
if (position == k) {
position = 1;
current = current->next;
} else {
position++;
current = current->next;
}
if (current == head) { // 如果回到起点,结束循环并返回次数
break;
}
}
return position;
}
int main() {
int k, data[5] = {1, 2, 3, 4, 5};
Node* head = NULL;
for (int i = 0; i < sizeof(data) / sizeof(data[0]); ++i) {
insertNode(&head, data[i]);
}
k = 3;
printf("The number of jumps required is %d\n", josephus(head, k));
return 0;
}
```
在这个代码里,我们首先创建了一个单循环链表,然后实现了约瑟夫环的问题。`josephus`函数计算出跳到最后一个位置的跳跃次数。在`main`函数中,我们创建了数据数组并插入到链表中,最后调用`josephus`函数获取结果。
阅读全文
相关推荐
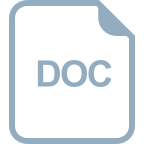
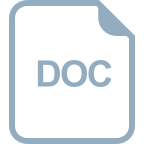
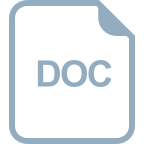
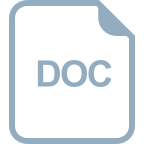

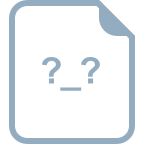
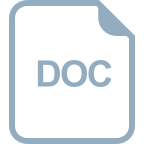
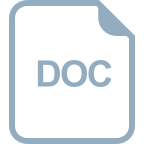
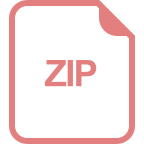
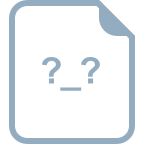
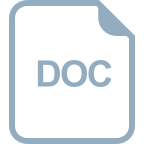
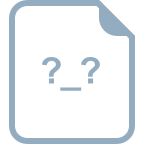
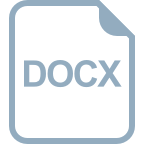
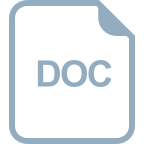
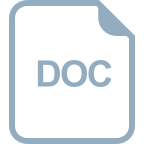
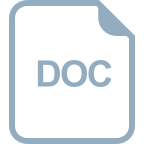
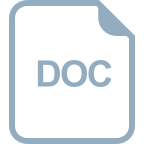
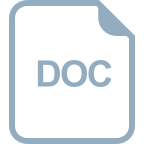