ajax增删改查项目源码
时间: 2024-12-27 18:17:03 浏览: 5
### Ajax 实现 CRUD 操作的项目源码示例
#### 创建 (Create)
为了通过 AJAX 向服务器发送创建请求,可以使用如下 JavaScript 代码:
```javascript
function createRecord(data) {
fetch('/api/records', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(data),
})
.then(response => response.json())
.then(result => console.log('Success:', result))
.catch(error => console.error('Error:', error));
}
```
此函数用于向 `/api/records` 发送 POST 请求来创建新记录[^1]。
#### 查询 (Read)
查询操作可以通过 GET 方法获取数据列表:
```javascript
function readRecords() {
fetch('/api/records')
.then(response => response.json())
.then(data => displayData(data)) // 假设有一个名为displayData的方法用来显示数据
.catch(error => console.error('Error fetching data:', error));
}
```
这段脚本会调用 API 获取所有记录并将其传递给 `displayData()` 函数处理[^2]。
#### 更新 (Update)
更新现有条目时可采用 PUT 或 PATCH 方法。这里展示如何利用 PUT 来修改特定 ID 的项:
```javascript
function updateRecord(id, updatedFields) {
fetch(`/api/record/${id}`, {
method: 'PUT',
headers: {'Content-Type': 'application/json'},
body: JSON.stringify(updatedFields)
})
.then(response => response.json())
.then(() => alert('Item successfully updated'))
.catch(error => console.error('Failed to update item:', error));
}
```
上述代码片段展示了怎样针对指定ID执行更新动作[^3]。
#### 删除 (Delete)
最后是删除功能,这通常涉及 DELETE HTTP 动词的应用程序逻辑:
```javascript
function deleteRecord(id) {
fetch(`/api/record/${id}`, {
method: 'DELETE'
})
.then(response => {
if (!response.ok) throw new Error('Network response was not ok');
return response;
})
.then(() => alert(`Deleted record with id ${id}`))
.catch(error => console.error('There has been a problem with your fetch operation:', error));
}
```
该部分实现了移除单个数据库条目的能力[^4]。
阅读全文
相关推荐










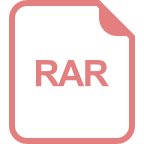







