基于栈的中缀算术表达式求值
时间: 2023-11-10 08:01:14 浏览: 172
基于栈的中缀算术表达式求值是一种计算中缀表达式的方法。这种方法通过维护两个栈,一个操作数栈和一个运算符栈,来按照运算符的优先级进行计算。具体步骤如下:
1. 创建一个空的操作数栈和一个空的运算符栈。
2. 从左到右遍历中缀表达式的每个字符。
3. 如果当前字符是数字,则将其解析为一个操作数并将其压入操作数栈。
4. 如果当前字符是运算符,并且运算符栈为空,或者当前运算符的优先级高于或等于运算符栈顶运算符的优先级,则将当前运算符压入运算符栈。
5. 如果当前字符是运算符,并且当前运算符的优先级低于运算符栈顶运算符的优先级,则从运算符栈弹出栈顶运算符,从操作数栈弹出两个操作数进行计算,然后将计算结果压入操作数栈。重复此过程直到当前运算符的优先级大于或等于运算符栈顶运算符的优先级,然后将当前运算符压入运算符栈。
6. 如果当前字符是左括号,则将其压入运算符栈。
7. 如果当前字符是右括号,则从运算符栈中弹出运算符,从操作数栈中弹出两个操作数进行计算,然后将计算结果压入操作数栈。重复此过程直到遇到左括号为止。
8. 遍历完整个中缀表达式后,从运算符栈中依次弹出运算符,从操作数栈中依次弹出两个操作数进行计算,然后将计算结果压入操作数栈。
9. 最后,操作数栈中的唯一元素即为中缀表达式的计算结果。
相关问题
基于栈的中缀算术表达式求值cpp
基于栈的中缀算术表达式求值通常是通过逆波兰表示法(Reverse Polish Notation, RPN)来实现的,因为栈数据结构非常适合处理这种操作。在C++中,你可以按照以下步骤进行:
1. **输入转换**:首先,你需要将用户输入的中缀表达式转化为RPN。这通常涉及两个栈:一个用于存储操作数,另一个用于存储运算符。遇到操作数就直接压入操作数栈,遇到运算符则比较其优先级,如果当前栈顶运算符优先级低于新运算符,则依次弹出操作数和运算符,直到找到等于或高于新运算符的,然后将新运算符压入栈中。
2. **计算过程**:遍历操作数堆栈,每次取出两个元素,一个是操作数,另一个是运算符。对这两个操作数应用运算符,并把结果推回栈上。这个过程会一直持续到只剩下一个元素为止,即得到最终的结果。
3. **返回结果**:最后的操作数就是表达式的值。
下面是一个简单的示例代码片段,展示了基本的思路:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <cctype>
int precedence(char op) {
// 定义运算符的优先级
return op == '+' || op == '-' ? 1 : 2;
}
bool isOperator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
std::string infixToRPN(std::string expr) {
std::stack<char> ops;
std::string result;
for (char ch : expr) {
if (isdigit(ch)) {
result += ch; // 操作数直接添加到结果中
} else if (isOperator(ch)) {
while (!ops.empty() && precedence(ops.top()) >= precedence(ch)) {
result += ops.top();
ops.pop();
}
ops.push(ch);
} else if (ch == '(') {
ops.push(ch);
} else if (ch == ')') {
while (ops.top() != '(') {
result += ops.top();
ops.pop();
}
ops.pop(); // 弹出左括号
}
}
while (!ops.empty()) {
result += ops.top();
ops.pop();
}
return result;
}
int evaluateRPN(std::string rpn) {
std::stack<int> stack;
for (char ch : rpn) {
if (isdigit(ch)) {
stack.push(ch - '0'); // 将字符转为数字并压入栈
} else {
int right = stack.top(); stack.pop();
int left = stack.top(); stack.pop();
switch (ch) {
case '+': stack.push(left + right); break;
case '-': stack.push(left - right); break;
case '*': stack.push(left * right); break;
case '/': stack.push(left / right); break;
}
}
}
return stack.top(); // 返回最终结果
}
int main() {
std::string expr = "4 + 5 * (7 - 9) / 2";
std::string rpn = infixToRPN(expr);
int result = evaluateRPN(rpn);
std::cout << "Result: " << result << std::endl;
return 0;
}
```
基于栈的中缀算术表达式求值c语言
基于栈的数据结构可以在C语言中用于计算中缀表达式的值,也称为逆波兰表示法(RPN)。这种技术利用了栈的特点:后进先出(LIFO)。以下是基本步骤:
1. **输入处理**:遍历中缀表达式的每个字符,如果是数字则压入栈,如果是运算符则执行操作。
2. **运算符处理**:
- 如果遇到运算符,取出栈顶两个元素(如果有两个),进行相应的运算(如加、减、乘、除),并将结果替换这两个元素,然后将运算结果放回栈顶。
- 如果栈顶只有一个元素,或者遇到优先级更低的运算符,则直接将当前运算符压入栈。
3. **结束处理**:当遍历完所有字符后,栈顶剩余的就是最终的结果。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
char op; // 运算符
} Token;
int isOperator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/');
}
double applyOp(double a, double b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': if (b != 0) return a / b; else return 0; // 防止除数为零
}
}
double evaluateRPN(char* tokens) {
int length = strlen(tokens);
Token stack[length]; // 栈大小等于表达式长度
int top = -1; // 栈顶指针初始化为-1
for (int i = 0; i < length; ++i) {
if (!isOperator(tokens[i])) { // 数字
stack[++top].op = tokens[i] - '0'; // 把数字转换成整数并压入栈
} else { // 运算符
while (top >= 0 && isOperator(stack[top].op)) { // 找到最近的左括号
double val2 = stack[top].op;
top--;
double val1 = stack[top].op;
stack[top].op = applyOp(val1, val2, stack[top].op); // 计算并更新栈顶元素
}
stack[++top].op = tokens[i];
}
}
// 最后的两个元素是结果
return applyOp(stack[top].op, stack[top - 1].op, stack[top].op);
}
int main() {
char expr[] = "2 3 + 4 *";
printf("Result: %.2f\n", evaluateRPN(expr));
return 0;
}
```
阅读全文
相关推荐








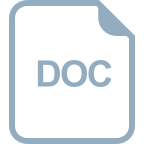

