使用C++语言实现简化RSA: ①实现简化RSA的加解密。 ②输入足够长度明文,例如1024字节的明文,实现其加解密。 ③使用openssl。
时间: 2024-10-11 08:14:35 浏览: 62
在C++中实现简化版的RSA加密算法通常包括以下几个步骤:
1. **生成公钥和私钥**:
- RSA算法基于大质数分解的困难性。首先,选择两个大素数p和q,计算n=p*q作为模数,然后取欧拉函数φ(n)=(p-1)*(q-1)的乘积作为欧拉 totient。
- 然后选择一个e(小于φ(n),且与φ(n)互质),用于构造公钥(e,n),公钥的主要作用是加密信息。
- 私钥d的求解涉及到扩展欧几里得算法,它满足(d*e)%φ(n)=1。
2. **加密(公钥加密)**:
- 对明文m(需要小于n)应用模指数运算,即c = m^e mod n,得到加密后的密文c。
3. **解密(私钥解密)**:
- 解密过程是逆向的,使用私钥d,通过m = c^d mod n计算出原始明文m。
4. **利用OpenSSL**:
OpenSSL是一个强大的安全套接字层(SSL/TLS)库,可以方便地处理RSA操作。你可以使用它的`EVP_PKEY_encrypt()`和`EVP_PKEY_decrypt()`函数来实现加密和解密。示例代码可能如下:
```cpp
#include <openssl/pem.h>
#include <openssl/rsa.h>
// 加密部分
RSA* rsa_pubkey;
int encrypted_len;
unsigned char encrypted_data[1024];
// 假设已经加载了公钥PEM文件
RSA* load_rsa_pubkey(const std::string& pem_file) {
// ...
}
std::string encrypt(const std::string& plaintext, const RSA* pubkey) {
int decrypted_len = RSA_size(pubkey);
unsigned char* decrypted_data = new unsigned char[decrypted_len];
int ret = RSA_public_encrypt(strlen(plaintext), (const unsigned char*)plaintext.c_str(), decrypted_data, pubkey, RSA_PKCS1_OAEP_PADDING);
if (ret == -1) {
// 处理错误...
}
encrypted_data = decrypted_data; // 保存加密结果
// 返回加密数据
// ...
}
// 解密部分
std::string decrypt(const unsigned char* ciphertext, RSA* privkey) {
int decrypted_len = RSA_size(privkey);
std::string plaintext(decrypted_len, '\0');
int ret = RSA_private_decrypt(decrypted_len, ciphertext, (char*)&plaintext[0], privkey, RSA_PKCS1_OAEP_PADDING);
// 处理错误...
return plaintext.substr(0, ret); // 返回解密后的明文
}
```
注意,上述代码仅作为一个简化示例,并未处理所有的错误情况和内存管理。实际使用时需要更完善的错误检查和资源释放。
阅读全文
相关推荐
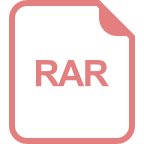
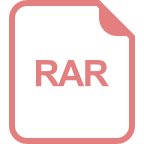
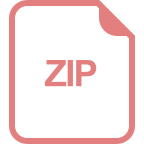

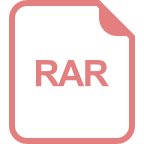
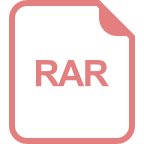
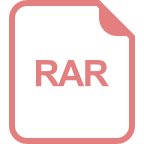
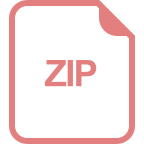
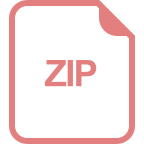
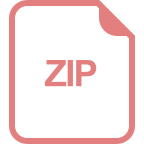
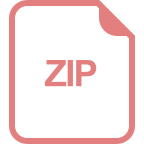
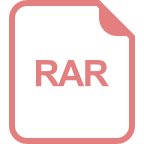
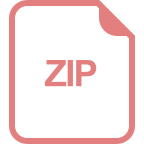
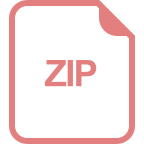
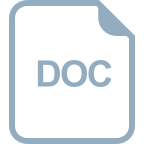
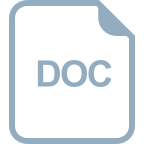


