如何把编码好的h264实时流 通过FFmpeg 推流 代码 FFmpeg版本4.2
时间: 2024-02-04 18:09:53 浏览: 201
要将编码好的H264实时流通过FFmpeg推流,你可以使用以下步骤:
1. 首先,确保你已经安装了FFmpeg,并且版本是4.2或更高版本。
2. 创建一个FFmpeg的输入上下文(AVFormatContext),用于读取H264实时流。你可以使用avformat_alloc_context()函数来分配上下文对象。
3. 设置输入上下文的参数,包括输入流的URL、格式和其他相关参数。你可以使用avformat_open_input()函数来打开输入流。
4. 查找并打开输入流中的视频解码器。你可以使用avformat_find_stream_info()函数来获取流信息,并使用avcodec_find_decoder()函数来查找解码器。
5. 创建一个FFmpeg的输出上下文(AVFormatContext),用于推送流。你可以使用avformat_alloc_output_context2()函数来分配上下文对象。
6. 设置输出上下文的参数,包括输出流的URL、格式和其他相关参数。你可以使用avformat_new_stream()函数来创建一个新的输出流。
7. 查找并打开输出流中的视频编码器。你可以使用avcodec_find_encoder()函数来查找编码器,并使用avcodec_open2()函数来打开编码器。
8. 使用avio_open2()函数打开输出URL,并使用avformat_write_header()函数写入输出文件头。
9. 循环读取输入流中的数据包,解码并编码为H264格式,然后写入输出流中。你可以使用av_read_frame()函数读取数据包,使用avcodec_send_packet()函数发送数据包给解码器,使用avcodec_receive_frame()函数接收解码后的帧,使用avcodec_send_frame()函数发送帧给编码器,使用avcodec_receive_packet()函数接收编码后的数据包,最后使用av_write_frame()函数写入输出流。
10. 在推流完成后,使用av_write_trailer()函数写入输出文件尾,并释放所有的上下文和资源。
下面是一个简单的示例代码,演示了如何将编码好的H264实时流通过FFmpeg推流:
```c
#include <stdio.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
int main() {
// 输入流参数
const char* input_url = "input.h264";
AVFormatContext* input_ctx = NULL;
AVCodecContext* input_codec_ctx = NULL;
AVCodec* input_codec = NULL;
// 输出流参数
const char* output_url = "rtmp://example.com/live/stream";
AVFormatContext* output_ctx = NULL;
AVCodecContext* output_codec_ctx = NULL;
AVCodec* output_codec = NULL;
// 打开输入流
avformat_open_input(&input_ctx, input_url, NULL, NULL);
avformat_find_stream_info(input_ctx, NULL);
avcodec_parameters_to_context(input_codec_ctx, input_ctx->streams[0]->codecpar);
input_codec = avcodec_find_decoder(input_codec_ctx->codec_id);
avcodec_open2(input_codec_ctx, input_codec, NULL);
// 打开输出流
avformat_alloc_output_context2(&output_ctx, NULL, "flv", output_url);
output_codec = avcodec_find_encoder(AV_CODEC_ID_H264);
output_codec_ctx = avcodec_alloc_context3(output_codec);
output_codec_ctx->width = input_codec_ctx->width;
output_codec_ctx->height = input_codec_ctx->height;
output_codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
avcodec_open2(output_codec_ctx, output_codec, NULL);
AVStream* output_stream = avformat_new_stream(output_ctx, output_codec);
avcodec_parameters_from_context(output_stream->codecpar, output_codec_ctx);
// 打开输出URL
avio_open2(&output_ctx->pb, output_url, AVIO_FLAG_WRITE, NULL);
avformat_write_header(output_ctx, NULL);
// 推流循环
AVPacket packet;
while (av_read_frame(input_ctx, &packet) >= 0) {
if (packet.stream_index == 0) {
avcodec_send_packet(input_codec_ctx, &packet);
AVFrame* frame = av_frame_alloc();
avcodec_receive_frame(input_codec_ctx, frame);
avcodec_send_frame(output_codec_ctx, frame);
av_packet_unref(&packet);
while (avcodec_receive_packet(output_codec_ctx, &packet) == 0) {
av_write_frame(output_ctx, &packet);
av_packet_unref(&packet);
}
av_frame_free(&frame);
}
}
// 写入文件尾
av_write_trailer(output_ctx);
// 释放资源
avformat_close_input(&input_ctx);
avcodec_free_context(&input_codec_ctx);
avformat_free_context(input_ctx);
avio_closep(&output_ctx->pb);
avcodec_free_context(&output_codec_ctx);
avformat_free_context(output_ctx);
return 0;
}
```
请注意,这只是一个简单的示例代码,实际应用中可能需要根据具体情况进行适当的修改和错误处理。
阅读全文
相关推荐


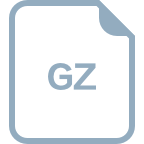













