用python做一个凯撒密码解密程序
时间: 2024-10-25 19:16:19 浏览: 19
凯撒密码是一种简单的替换加密方法,通过将明文中的每个字母按照固定的位移进行替换来达到加密的目的。下面是一个简单的Python程序,用于解密凯撒密码:
```python
def caesar_cipher_decrypt(ciphertext, shift):
plaintext = ""
for char in ciphertext:
if char.isalpha():
# 对大写字母和小写字母分别处理
shift_amount = shift % 26
if char.islower():
new_char = chr(((ord(char) - ord('a') - shift_amount) % 26) + ord('a'))
else:
new_char = chr(((ord(char) - ord('A') - shift_amount) % 26) + ord('A'))
plaintext += new_char
else:
plaintext += char # 非字母字符保持原样
return plaintext
# 示例用法
ciphertext = "Khoor, Zruog!"
shift = 3 # 假设加密位移为3 (实际可根据需要调整)
decrypted_text = caesar_cipher_decrypt(ciphertext, shift)
print(f"原始文本: {decrypted_text}")
```
在这个程序中,`caesar_cipher_decrypt`函数接收两个参数:密文(`ciphertext`)和偏移量(`shift`)。它遍历密文中的每个字符,如果是字母,则根据ASCII码进行相应的位移并转换回对应的字母;如果不是字母,则直接保留不变。
阅读全文
相关推荐
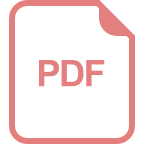
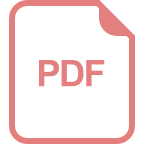
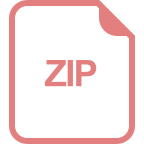









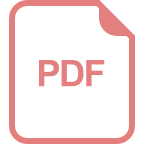





