LSTM多输入多输出pytorch
时间: 2023-10-30 13:05:52 浏览: 63
在PyTorch中,你可以使用LSTM模型进行多输入多输出的任务。下面是一个简单的示例代码,演示如何使用LSTM模型实现多输入多输出任务。
首先,导入所需的库:
```python
import torch
import torch.nn as nn
```
然后定义LSTM模型类:
```python
class LSTMModel(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(LSTMModel, self).__init__()
self.hidden_size = hidden_size
self.lstm = nn.LSTM(input_size, hidden_size)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, input1, input2):
_, (hidden, _) = self.lstm(input1)
output = self.fc(hidden[-1])
return output
```
在上述代码中,我们定义了一个包含一个LSTM层和一个全连接层的模型。输入数据input1和input2分别作为模型的两个输入。在forward()方法中,我们只使用了input1,并通过LSTM层和全连接层获得输出。你可以根据自己的任务需求进行修改。
接下来,实例化模型并定义输入数据:
```python
input1 = torch.randn(10, 1, 1) # input1的形状为(batch_size, sequence_length, input_size)
input2 = torch.randn(10, 1, 1) # input2的形状为(batch_size, sequence_length, input_size)
model = LSTMModel(input_size=1, hidden_size=16, output_size=1)
```
最后,使用模型进行前向传播计算:
```python
output = model(input1, input2)
print(output)
```
以上代码展示了如何使用LSTM模型实现多输入多输出的任务。你可以根据自己的需求修改模型的结构和输入数据的形状。希望对你有所帮助!
相关推荐
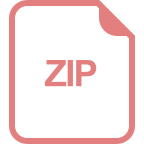














