c++调用opencv写成dll
时间: 2024-10-14 17:05:11 浏览: 33
在C++中利用OpenCV编写DLL(动态链接库)主要是为了将OpenCV的功能模块暴露给其他应用程序使用。以下是简单的步骤:
1. **安装和配置**:
- 首先,确保已经安装了OpenCV库及其对应的C++开发工具包(例如OpenCV for Visual Studio)。
- 安装完成后,设置环境变量以指向包含头文件和库文件的路径。
2. **创建源代码**:
- 创建一个新的C++项目,然后添加一个源文件,例如`MyOpencvDll.cpp`。在这个文件中,你需要声明公开函数,它们通常接收OpenCV的数据结构作为输入,并可能返回结果。
```cpp
#include "opencv2/opencv.hpp"
extern "C" __declspec(dllexport) cv::Mat processImage(cv::Mat input) {
// 在这里实现图像处理逻辑
cv::Mat result = cv::Mat::zeros(input.size(), input.type());
// ... 对input做处理
return result;
}
```
3. **编译为DLL**:
- 设置项目的构建目标为动态库(如Visual Studio中选择“Win32 Dynamic-Link Library”或`.lib`为输出文件名)。
- 编译过程中记得链接OpenCV库,如`opencv_core.lib`, `opencv_highgui.lib`等。
4. **使用DLL**:
- 将生成的DLL文件放在应用程序的搜索路径下,或者明确指定其位置。
- 在需要使用OpenCV功能的应用程序中,通过动态链接的方式调用DLL中的函数。
```cpp
#include <windows.h>
#include <opencv2/opencv.hpp>
cv::Mat getImage() {
HMODULE hModule = LoadLibrary("path_to_your_dll.dll");
if (hModule == nullptr) {
std::cerr << "Failed to load DLL" << std::endl;
return {};
}
// 获取函数指针
auto processImageFn = (processImageProc)GetProcAddress(hModule, "processImage");
if (processImageFn == nullptr) {
std::cerr << "Function not found in the DLL" << std::endl;
FreeLibrary(hModule);
return {};
}
cv::Mat inputImage = cv::imread("image.jpg");
cv::Mat processedImage = processImageFn(inputImage);
FreeLibrary(hModule); // 可选,释放DLL资源
return processedImage;
}
阅读全文
相关推荐
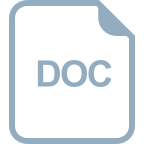
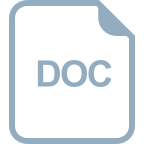
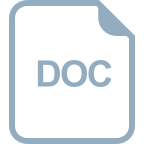

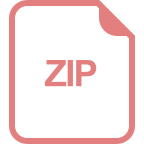
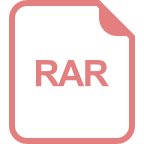
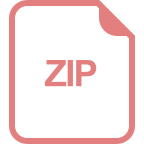
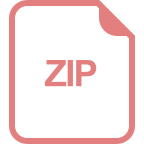
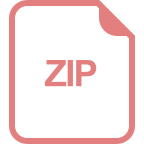







