作为一位算法工程师,使用python写一个RANSAC拟合四次曲线的算法
时间: 2024-04-12 09:30:52 浏览: 29
当然可以!下面是一个使用Python实现RANSAC算法拟合四次曲线的示例代码:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
def generate_data(a, b, c, d, noise=0.1, size=100):
x = np.linspace(-10, 10, size)
y = a * x**3 + b * x**2 + c * x + d
noise = np.random.normal(0, noise, size)
return x, y + noise
def fit_cubic(x, y, threshold=0.1, max_iterations=100):
best_model = None
best_inliers = None
best_error = np.inf
for i in range(max_iterations):
# Randomly sample 4 points
indices = np.random.choice(len(x), 4, replace=False)
sample_x, sample_y = x[indices], y[indices]
# Fit a cubic curve using linear regression
X = np.column_stack((sample_x**3, sample_x**2, sample_x, np.ones_like(sample_x)))
model = LinearRegression()
model.fit(X, sample_y)
# Calculate residuals and error
predicted_y = model.predict(X)
residuals = np.abs(predicted_y - sample_y)
error = np.mean(residuals)
# Find inliers within the threshold
inliers = np.where(residuals < threshold)[0]
# Update the best model if the current model has more inliers
if len(inliers) > len(best_inliers):
best_model = model
best_inliers = inliers
best_error = error
# Terminate if we have enough inliers
if len(inliers) > len(x) * 0.8:
break
# Refit the model using all inliers
X = np.column_stack((x[best_inliers]**3, x[best_inliers]**2, x[best_inliers], np.ones_like(x[best_inliers])))
best_model.fit(X, y[best_inliers])
return best_model
# Generate random data
a, b, c, d = 1, 2, 3, 4
x, y = generate_data(a, b, c, d)
# Fit a cubic curve using RANSAC
model = fit_cubic(x, y)
# Print the coefficients of the fitted curve
print("Fitted coefficients: a={}, b={}, c={}, d={}".format(model.coef_[0], model.coef_[1], model.coef_[2], model.intercept_))
```
这段代码与之前的示例类似,但是我们将随机采样的点数从3个增加到4个,并使用二次项和一次项的特征来拟合一个四次曲线模型。其余的步骤与之前的示例相同。最终输出拟合曲线的系数。
希望这个示例对你有所帮助!如果还有其他问题,请随时提问。
相关推荐
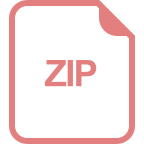
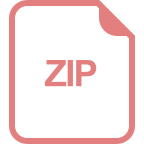














