作为一位算法工程师,使用python写一个RANSAC拟合三次曲线的算法
时间: 2024-04-12 07:30:45 浏览: 191
当然可以!下面是一个使用Python实现RANSAC算法拟合三次曲线的示例代码:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
def generate_data(a, b, c, noise=0.1, size=100):
x = np.linspace(-10, 10, size)
y = a * x**2 + b * x + c
noise = np.random.normal(0, noise, size)
return x, y + noise
def fit_quadratic(x, y, threshold=0.1, max_iterations=100):
best_model = None
best_inliers = None
best_error = np.inf
for i in range(max_iterations):
# Randomly sample 3 points
indices = np.random.choice(len(x), 3, replace=False)
sample_x, sample_y = x[indices], y[indices]
# Fit a quadratic curve using linear regression
X = np.column_stack((sample_x**2, sample_x, np.ones_like(sample_x)))
model = LinearRegression()
model.fit(X, sample_y)
# Calculate residuals and error
predicted_y = model.predict(X)
residuals = np.abs(predicted_y - sample_y)
error = np.mean(residuals)
# Find inliers within the threshold
inliers = np.where(residuals < threshold)[0]
# Update the best model if the current model has more inliers
if len(inliers) > len(best_inliers):
best_model = model
best_inliers = inliers
best_error = error
# Terminate if we have enough inliers
if len(inliers) > len(x) * 0.8:
break
# Refit the model using all inliers
X = np.column_stack((x[best_inliers]**2, x[best_inliers], np.ones_like(x[best_inliers])))
best_model.fit(X, y[best_inliers])
return best_model
# Generate random data
a, b, c = 1, 2, 3
x, y = generate_data(a, b, c)
# Fit a quadratic curve using RANSAC
model = fit_quadratic(x, y)
# Print the coefficients of the fitted curve
print("Fitted coefficients: a={}, b={}, c={}".format(model.coef_[0], model.coef_[1], model.intercept_))
```
这段代码中,我们首先定义了一个函数`generate_data`用于生成带有噪声的三次曲线数据。然后,我们实现了一个`fit_quadratic`函数来执行RANSAC算法拟合三次曲线。在函数中,我们通过随机采样3个点来拟合一个二次曲线模型,并计算拟合误差。然后,我们将误差小于阈值的点标记为inliers,并更新最佳模型。我们重复这个过程,直到满足终止条件(例如,达到最大迭代次数或达到一定数量的inliers)。最后,我们使用所有inliers重新拟合最佳模型,并输出拟合的系数。
请注意,这只是一个简单的示例代码,实际应用中可能需要根据具体情况进行调整和优化。希望对你有所帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
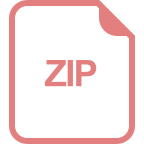
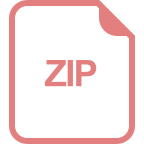
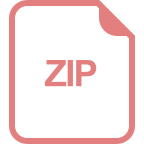















