使用opencv利用c#在unity里实现最邻近差值调整图像大小代码
时间: 2024-04-14 13:31:06 浏览: 11
在 Unity 中使用 C# 和 OpenCV 来实现最邻近差值调整图像大小的代码可以参考以下示例:
```csharp
using UnityEngine;
using OpenCvSharp;
public class ImageResizer : MonoBehaviour
{
public Texture2D inputTexture;
public int outputWidth = 512;
public int outputHeight = 512;
private Texture2D outputTexture;
void Start()
{
// 将输入的 Unity 纹理转换为 OpenCV 图像
Mat inputImage = UnityTextureToMat(inputTexture);
// 调整图像大小
Mat resizedImage = new Mat();
Cv2.Resize(inputImage, resizedImage, new Size(outputWidth, outputHeight), 0, 0, InterpolationFlags.Nearest);
// 将调整后的图像转换为 Unity 纹理
outputTexture = MatToUnityTexture(resizedImage);
// 将调整后的图像显示在屏幕上
Renderer renderer = GetComponent<Renderer>();
renderer.material.mainTexture = outputTexture;
}
private Mat UnityTextureToMat(Texture2D texture)
{
Mat mat = new Mat(texture.height, texture.width, MatType.CV_8UC3);
Color32[] colors = texture.GetPixels32();
byte[] data = new byte[texture.width * texture.height * 3];
int index = 0;
for (int y = 0; y < texture.height; y++)
{
for (int x = 0; x < texture.width; x++)
{
Color32 color = colors[y * texture.width + x];
data[index++] = color.r;
data[index++] = color.g;
data[index++] = color.b;
}
}
mat.SetArray(0, 0, data);
return mat;
}
private Texture2D MatToUnityTexture(Mat mat)
{
Texture2D texture = new Texture2D(mat.Cols, mat.Rows, TextureFormat.RGB24, false);
byte[] data = new byte[mat.Cols * mat.Rows * 3];
mat.GetArray(0, 0, data);
Color32[] colors = new Color32[mat.Cols * mat.Rows];
int index = 0;
for (int y = 0; y < mat.Rows; y++)
{
for (int x = 0; x < mat.Cols; x++)
{
colors[index++] = new Color32(data[index], data[index + 1], data[index + 2], 255);
index += 3;
}
}
texture.SetPixels32(colors);
texture.Apply();
return texture;
}
}
```
以上代码会将输入的纹理 `inputTexture` 调整为指定的大小 `outputWidth` 和 `outputHeight`,并在 Unity 中显示调整后的图像。注意,需要在 Unity 中安装并引用 OpenCvSharp 库。
相关推荐
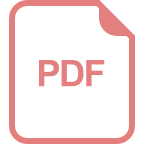














