循环队列表输出C语言
时间: 2024-10-13 18:08:14 浏览: 6
循环队列是一种特殊的线性表数据结构,它在数组的一端插入元素,在另一端删除元素。它的特点是队列的两端可以同时作为头结点和尾结点使用,通过循环机制实现高效的插入和删除操作。
在C语言中,我们可以使用数组来实现循环队列。这里有一个基本的循环队列结构体定义和相关操作:
```c
#include <stdio.h>
#define MAX_SIZE 10
typedef struct {
int *queue; // 存储数据的数组
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化循环队列
void initQueue(Queue *q) {
q->queue = (int*)malloc(MAX_SIZE * sizeof(int));
if (!q->queue) {
printf("Memory allocation failed.\n");
return;
}
q->front = q->rear = -1;
}
// 入队操作
void enqueue(Queue *q, int value) {
if ((q->rear + 1) % MAX_SIZE == q->front) { // 当队列满时处理溢出
printf("Queue is full.\n");
return;
}
q->rear = (q->rear + 1) % MAX_SIZE; // 向后移动队尾
q->queue[q->rear] = value;
}
// 出队操作
int dequeue(Queue *q) {
if (q->front == -1) { // 当队列为空时处理异常
printf("Queue is empty.\n");
return -1;
}
int value = q->queue[q->front]; // 获取队头元素
q->front = (q->front + 1) % MAX_SIZE; // 移动队头
return value;
}
// 打印队列内容
void printQueue(Queue *q) {
if (q-1) {
printf("Queue is empty.\n");
return;
}
for (int i = q->front; i != q->rear; i = (i + 1) % MAX_SIZE) {
printf("%d ", q->queue[i]);
}
printf("\n");
}
int main() {
Queue queue;
initQueue(&queue);
// 添加一些元素并打印
enqueue(&queue, 1);
enqueue(&queue, 2);
enqueue(&queue, 3);
printQueue(&queue);
// 删除元素并打印剩余
dequeue(&queue);
printQueue(&queue);
return 0;
}
```
在这个例子中,我们创建了一个`Queue`结构体,包含指向存储数组的指针、队头和队尾指针。`enqueue`和`dequeue`函数实现了入队和出队操作,而`printQueue`用于显示队列的内容。在`main`函数中展示了循环队列的基本使用。
相关推荐
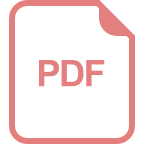
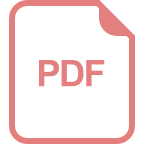
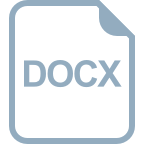















