算法设计题 以二叉链表作为二叉树的存储结构,编写以下算法: (1)统计二叉树的叶结点个数。 ‘2)用按层次顺序遍历二叉树的方法,统计树中具有度为1的结点数日。
时间: 2024-11-23 14:07:08 浏览: 32
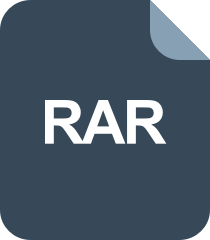
erchashubianli.rar_用二叉链表 进行 二叉树 遍历
对于这两个算法,我们需要分别处理二叉链表表示的二叉树:
1. **统计二叉树的叶节点个数**:
叶节点是没有子节点的节点,我们可以采用深度优先搜索(DFS)或广度优先搜索(BFS)。在BFS中,因为是从根节点开始逐层遍历,所以可以直接在遍历的过程中记录遇到的叶节点计数。从头节点开始,如果当前节点为空或其右孩子为空,说明是叶节点,增加计数。
```python
def countLeaves(root):
if not root:
return 0
queue = [root]
leaf_count = 0
while queue:
node = queue.pop(0)
if not node.left and not node.right:
leaf_count += 1
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return leaf_count
```
2. **统计树中具有度为1的节点数**:
度为1的节点意味着该节点只有一个子节点。同样,我们可以在BFS遍历时检查每个节点的子节点数量。如果一个节点只有一个子节点,那么它的度就是1。
```python
def countDegreeOneNodes(root):
if not root:
return 0
queue = [root]
degree_one_count = 0
while queue:
node = queue.pop(0)
if node.left and not node.right:
degree_one_count += 1
elif node.right and not node.left:
degree_one_count += 1
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return degree_one_count
```
以上两个函数分别实现了对二叉链表表示的二叉树的叶节点和度为1节点的计数。
阅读全文
相关推荐
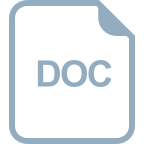
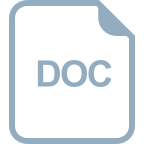










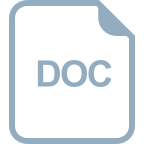
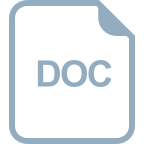
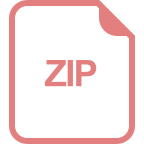
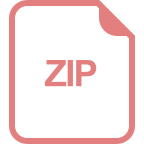