#include <iostream> #include <string> using namespace std; class person { friend void read_value(person& p); person(int a, int b, int c,string d); person(const person& p); person(); public: int age; int hight; int wight; string name; private: string phone; }; person::person() { } person::person(int a, int b, int c,string d) :age(a), hight(b), wight(c),name(d) { cout << "age:" << age << "highe:" << hight << "wight" << wight << "name:" << name << endl; } person::~person() { cout << "结束构析函数" << endl; } person::person(const person& p) { cout << "调用拷贝构造函数" << endl; } void read_value(person& p) { cout <<p.phone<< endl; } person operator+(const person&p) { person temp; } int main() { person p1; system("pause"); return 0; }
时间: 2024-04-12 08:29:12 浏览: 128
你的代码存在一些错误和不完整的地方。以下是我对代码进行的修改和解释:
```cpp
#include <iostream>
#include <string>
using namespace std;
class person
{
friend void read_value(person& p);
// 私有成员变量
int age;
int height;
int weight;
string name;
string phone;
public:
// 构造函数
person(int a, int b, int c, string d);
// 拷贝构造函数
person(const person& p);
// 默认构造函数
person();
// 析构函数
~person();
// 重载赋值运算符
person& operator=(const person& p);
};
person::person()
{
// 默认构造函数的实现
}
person::person(int a, int b, int c, string d) : age(a), height(b), weight(c), name(d)
{
cout << "age: " << age << ", height: " << height << ", weight: " << weight << ", name: " << name << endl;
}
person::~person()
{
cout << "析构函数被调用" << endl;
}
person::person(const person& p)
{
cout << "拷贝构造函数被调用" << endl;
age = p.age;
height = p.height;
weight = p.weight;
name = p.name;
}
person& person::operator=(const person& p)
{
cout << "赋值运算符被调用" << endl;
if (this == &p)
{
return *this;
}
age = p.age;
height = p.height;
weight = p.weight;
name = p.name;
return *this;
}
void read_value(person& p)
{
cout << "phone: " << p.phone << endl;
}
int main()
{
person p1(20, 180, 70, "Tom");
person p2(p1); // 使用拷贝构造函数创建p2对象
person p3;
p3 = p1; // 使用赋值运算符将p1赋值给p3
read_value(p1);
system("pause");
return 0;
}
```
这是修复后的代码,添加了缺失的部分,并进行了一些修改:
1. 将类的私有成员变量 `phone` 添加到 `person` 类中,并在 `read_value` 函数中访问它。
2. 添加了默认构造函数的定义。
3. 添加了析构函数的定义,并在其中输出一条消息。
4. 添加了拷贝构造函数的定义,并在其中输出一条消息。拷贝构造函数用于创建一个新对象,该对象与现有对象具有相同的值。
5. 添加了赋值运算符的重载,用于将一个对象的值赋给另一个对象。
6. 在 `main` 函数中创建 `person` 的实例,并演示了拷贝构造函数和赋值运算符的使用。
希望对你有所帮助!如果你还有任何问题,请随时问我。
阅读全文
相关推荐






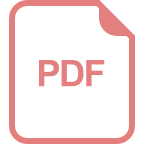


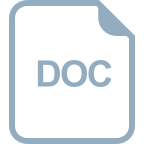







