用C++实现线性表的顺序表存储、链表存储、相关操作的编程实现;
时间: 2024-10-05 18:02:52 浏览: 53
在C++中,线性表可以使用顺序表(Array-Based List)和链表(Linked List)两种方式进行存储。
1. **顺序表(数组实现)**:
- 它使用一维动态数组来存储数据,每个元素都有一个连续的内存地址。
```cpp
#include <iostream>
using namespace std;
class SequentialList {
private:
int* data;
int size;
int capacity;
public:
SequentialList(int cap) {
data = new int[cap];
size = 0;
capacity = cap;
}
// 添加元素
void add(int value) {
if (size == capacity) resize(capacity * 2);
data[size++] = value;
}
// 删除元素
void remove(int index) {
if (index >= 0 && index < size) {
for (int i = index; i < size - 1; ++i)
data[i] = data[i + 1];
--size;
}
}
// 其他操作...
};
```
2. **链表(链式存储)**:
- 使用节点结构,每个节点包含数据和指向下一个节点的指针。
```cpp
#include <iostream>
struct Node {
int data;
Node* next;
};
class LinkedList {
private:
Node* head;
public:
LinkedList() { head = nullptr; }
// 添加元素
void add(int value) {
Node* newNode = new Node{value, nullptr};
if (!head) head = newNode;
else {
Node* current = head;
while (current->next) current = current->next;
current->next = newNode;
}
}
// 删除元素
void remove(int value) {
if (!head) return;
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next && current->next->data != value) {
current = current->next;
}
if (current->next) {
current->next = current->next->next;
delete current->next;
}
}
// 其他操作...
};
```
阅读全文
相关推荐
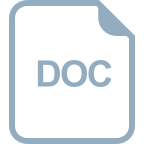
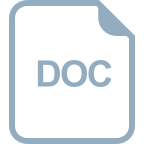
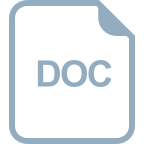


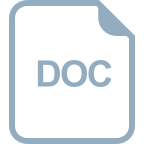


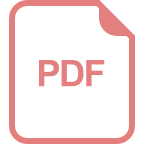
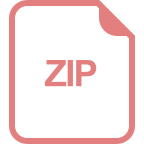
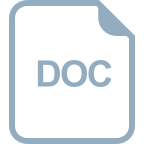
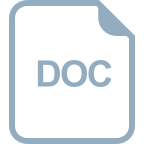
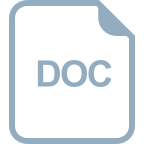
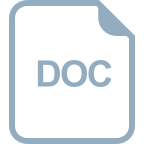
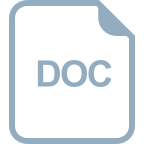
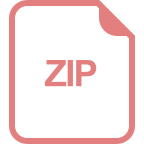
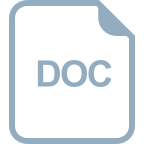
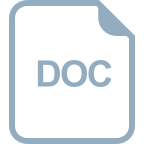
