如果我是在qtdesigner中已经画好了opengl widget我要怎么去调用它,用的是pyqt
时间: 2024-10-24 07:11:51 浏览: 45
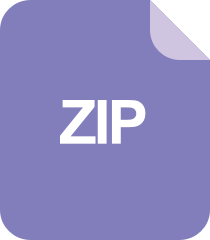
pyqt qt designer 实现音乐播放器界面设计
在Qt Designer中创建了一个OpenGL Widget之后,要在PyQt应用中实际使用它,你需要按照以下步骤进行:
1. **设计界面**:在Qt Designer中,选择并添加OpenGL Widget控件到你的用户界面布局中,并设置好其属性,如大小和位置。
2. **导出UI文件**:保存你的设计,生成`.ui`文件。你可以通过“File”菜单下的“Save As”选项,并选择“Qt Form Class (*.ui)”。
3. **编写Python代码**:打开Python编辑器,导入所需的模块:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget
from PyQt5 import uic
from OpenGL.GL import *
```
4. **加载UI**:使用`uic.loadUi()`函数加载`.ui`文件,将其转换为Python类对象:
```python
class MainWindow(QMainWindow):
def __init__(self):
super(MainWindow, self).__init__()
uic.loadUi('your_ui_file.ui', self)
```
5. **获取OpenGL Widget**:在`__init__`方法中,找到并存储你的OpenGL Widget的实例,通常名为`centralWidget`:
```python
self.centralWidget = self.findChild(QWidget, "your_opengl_widget_name")
```
6. **处理显示和事件**:创建一个OpenGL上下文,然后在需要的地方处理OpenGL渲染,例如在`paintEvent()`或专门的绘制函数中:
```python
def paintEvent(self, event):
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
# ... 进行OpenGL绘图操作 ...
self.update()
```
7. **运行应用程序**:最后,在主函数里创建`QApplication`,创建`MainWindow`实例,并启动应用:
```python
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐
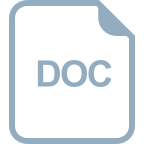
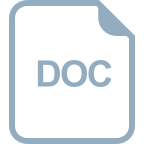

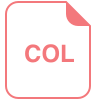













