python json读写操作
时间: 2024-04-02 16:29:55 浏览: 118
Python中可以使用内置的json模块进行JSON的读写操作。下面是一个简单的介绍:
1. JSON的读取:
- 使用`json.load()`函数可以从文件中读取JSON数据,并将其解析为Python对象。
- 使用`json.loads()`函数可以将JSON字符串解析为Python对象。
2. JSON的写入:
- 使用`json.dump()`函数可以将Python对象写入到文件中,以JSON格式保存。
- 使用`json.dumps()`函数可以将Python对象转换为JSON字符串。
下面是一个示例代码,演示了如何进行JSON的读写操作:
```python
import json
# 从文件中读取JSON数据
with open('data.json', 'r') as f:
data = json.load(f)
print(data)
# 将Python对象写入到文件中
data = {'name': 'John', 'age': 30, 'city': 'New York'}
with open('output.json', 'w') as f:
json.dump(data, f)
# 将Python对象转换为JSON字符串
data = {'name': 'John', 'age': 30, 'city': 'New York'}
json_str = json.dumps(data)
print(json_str)
```
相关问题
python jsonl读写
在Python中,JSONL(JSON Lines)是一种将JSON数据存储为行的形式,每一行对应一个独立的JSON对象。这种格式便于处理大量数据,因为它们可以方便地逐行读取和写入,而不必将整个文件加载到内存中。
**读取JSONL文件:**
使用`json`模块的`load`方法配合文件迭代器`open`,可以逐行读取JSONL文件中的内容:
```python
import json
def read_jsonl(file_path):
with open(file_path, 'r') as file:
for line in file:
json_data = json.loads(line)
# 处理每个json对象
process_json(json_data)
# 示例调用
read_jsonl('data.jsonl')
```
**写入JSONL文件:**
`json.dump`方法也可以用于逐行写入,只需要将每条数据包裹在`json.dumps`后的列表里:
```python
def write_jsonl(file_path, json_list):
with open(file_path, 'w') as file:
for data in json_list:
file.write(json.dumps(data) + '\n')
# 示例调用
data = [{"name": "Alice", "age": 30}, {"name": "Bob", "age": 35}]
write_jsonl('output.jsonl', data)
```
**相关问题--:**
1. JSONL格式的优点是什么?
2. 如果数据文件非常大,如何更有效地处理?
3. Python中有没有现成的库可以简化JSONL的读写操作?
python json文件读写
在Python中,可以使用内置的`json`模块来读写JSON文件。下面是一些常见的操作:
1. 读取JSON文件:
```python
import json
with open('data.json', 'r') as f:
data = json.load(f)
```
这里假设`data.json`是要读取的JSON文件。`json.load()`函数将文件内容加载为Python对象。
2. 写入JSON文件:
```python
import json
data = {'name': 'John', 'age': 30, 'city': 'New York'}
with open('data.json', 'w') as f:
json.dump(data, f)
```
这里创建了一个字典对象`data`,然后使用`json.dump()`函数将其写入到名为`data.json`的文件中。
3. 处理JSON数据:
读取后的JSON数据可以像操作Python对象一样进行处理。例如,可以通过键访问JSON对象的值:
```python
name = data['name']
age = data['age']
```
也可以在已有的JSON数据上进行修改,然后再写回到文件中。
请注意,在读写JSON文件时,需要确保文件存在并具有正确的JSON格式。否则,可能会抛出异常。
阅读全文
相关推荐
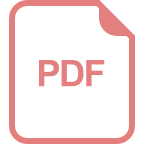
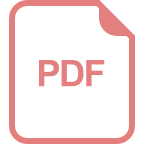
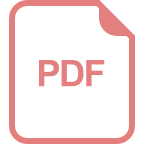












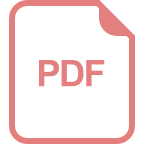
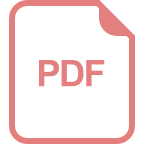