python的读写json
时间: 2024-02-12 08:01:46 浏览: 171
Python中读写JSON的操作非常简单,可以使用内置的json模块来实现。下面是一个简单的示例:
1. 读取JSON文件:
```python
import json
# 打开JSON文件
with open('data.json', 'r') as f:
# 加载JSON数据
data = json.load(f)
# 使用data变量访问JSON数据
print(data)
```
2. 写入JSON文件:
```python
import json
# 创建要写入的数据
data = {
'name': 'John',
'age': 30,
'city': 'New York'
}
# 打开JSON文件
with open('data.json', 'w') as f:
# 将数据写入文件
json.dump(data, f)
```
在上面的示例中,`json.load()`函数用于从文件中加载JSON数据并将其转换为Python对象,`json.dump()`函数用于将Python对象转换为JSON格式并写入文件。
相关问题
python 读写json
Python中读写JSON格式的数据可以使用内置的json模块,这里给出一些基本的示例代码。
1. 读取JSON文件
假设我们有一个名为data.json的JSON文件,其内容如下:
```json
{
"name": "John",
"age": 30,
"isMarried": true,
"hobbies": ["reading", "traveling", "sports"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
```
我们可以使用以下代码读取该文件并将其解析为Python对象:
```python
import json
with open('data.json', 'r') as f:
data = json.load(f)
print(data)
```
输出结果为:
```python
{
"name": "John",
"age": 30,
"isMarried": true,
"hobbies": ["reading", "traveling", "sports"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
```
2. 将Python对象写入JSON文件
假设我们有一个名为data的Python对象,其内容如下:
```python
data = {
"name": "John",
"age": 30,
"isMarried": True,
"hobbies": ["reading", "traveling", "sports"],
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY",
"zip": "10001"
}
}
```
我们可以使用以下代码将该Python对象写入名为output.json的JSON文件:
```python
import json
with open('output.json', 'w') as f:
json.dump(data, f)
```
这将在当前工作目录下创建一个名为output.json的文件,并将Python对象data的JSON表示写入其中。
以上就是基本的JSON读写操作,希望能对你有所帮助。
python读写json
读取JSON文件可以使用json模块的load函数来读取json文件,代码如下:
```python
import json
with open('data.json', 'r') as f:
data = json.load(f)
```
另外,也可以使用json模块的loads函数来读取json字符串,代码如下:
```python
import json
with open('data.json', 'r') as f:
data_str = f.read()
data = json.loads(data_str)
```
写入JSON文件可以使用json模块的dump函数来写入json文件,代码如下:
```python
import json
data = {'name': 'Tom', 'age': 18}
with open('data.json', 'w') as f:
json.dump(data, f)
```
另外,也可以使用json模块的dumps函数将字典转换为json字符串并写入文件,代码如下:
```python
import json
data = {'name': 'Tom', 'age': 18}
with open('data.json', 'w') as f:
data_str = json.dumps(data)
f.write(data_str)
```
以上是使用json模块进行读写json文件的方法。\[1\]\[2\]
#### 引用[.reference_title]
- *1* *2* [python读写json文件方法详解](https://blog.csdn.net/qq_42751978/article/details/130668983)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [Python读写json数据详解](https://blog.csdn.net/qq_37189082/article/details/124654767)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐
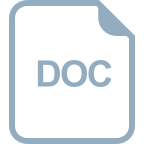
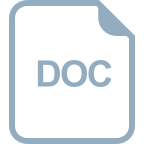
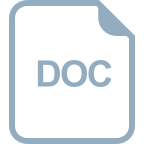
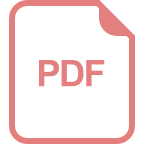
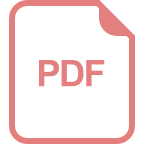




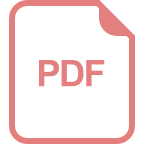
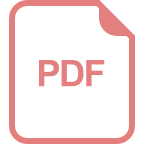


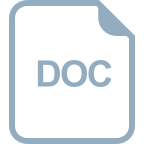
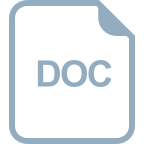
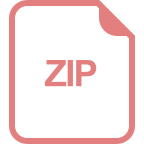