stm32f103矩阵键盘程序4*4详细代码
时间: 2023-07-26 11:05:00 浏览: 384
下面是一个基于STM32F103的4x4矩阵键盘程序的详细代码示例:
```c
#include "stm32f10x.h"
#include "stdio.h"
GPIO_InitTypeDef GPIO_InitStructure;
// 定义键盘矩阵的行和列
#define ROWS 4
#define COLS 4
// 定义行引脚和列引脚
GPIO_TypeDef* ROW_PORT[ROWS] = { GPIOA, GPIOA, GPIOA, GPIOA };
uint16_t ROW_PIN[ROWS] = { GPIO_Pin_0, GPIO_Pin_1, GPIO_Pin_2, GPIO_Pin_3 };
GPIO_TypeDef* COL_PORT[COLS] = { GPIOA, GPIOA, GPIOA, GPIOA };
uint16_t COL_PIN[COLS] = { GPIO_Pin_4, GPIO_Pin_5, GPIO_Pin_6, GPIO_Pin_7 };
// 定义键盘矩阵按键对应的字符
char keymap[ROWS][COLS] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
void Delay(__IO uint32_t nCount)
{
for(; nCount != 0; nCount--);
}
void init_GPIO()
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置行引脚为上拉输入模式
for(int i = 0; i < ROWS; i++)
{
GPIO_InitStructure.GPIO_Pin = ROW_PIN[i];
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(ROW_PORT[i], &GPIO_InitStructure);
}
// 配置列引脚为推挽输出模式
for(int i = 0; i < COLS; i++)
{
GPIO_InitStructure.GPIO_Pin = COL_PIN[i];
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(COL_PORT[i], &GPIO_InitStructure);
}
}
char read_keypad()
{
for(int i = 0; i < COLS; i++)
{
// 将当前列引脚置低电平
GPIO_ResetBits(COL_PORT[i], COL_PIN[i]);
for(int j = 0; j < ROWS; j++)
{
// 读取当前行引脚状态
if(GPIO_ReadInputDataBit(ROW_PORT[j], ROW_PIN[j]) == Bit_RESET)
{
// 延时一段时间,防止按键抖动
Delay(10000);
// 再次检查按键状态
if(GPIO_ReadInputDataBit(ROW_PORT[j], ROW_PIN[j]) == Bit_RESET)
{
// 按键按下,返回对应字符
return keymap[j][i];
}
}
}
// 将当前列引脚恢复为高电平
GPIO_SetBits(COL_PORT[i], COL_PIN[i]);
}
// 没有按键按下,返回空字符
return '\0';
}
int main(void)
{
init_GPIO();
while(1)
{
char key = read_keypad();
if(key != '\0')
{
printf("Pressed key: %c\r\n", key);
}
// 延时一段时间,防止按键重复检测
Delay(500000);
}
}
```
这段代码使用了STM32F103的GPIO模块,通过设置行引脚为上拉输入模式和列引脚为推挽输出模式,实现了对4x4矩阵键盘的读取。通过对行引脚和列引脚逐个进行扫描,可以检测到按键的状态,并返回对应的字符。在主循环中,通过调用`read_keypad()`函数来读取按键,并将结果打印出来。
请注意,这只是一个示例代码,实际使用时可能需要根据具体的硬件连接和引脚配置进行适当的修改。
阅读全文
相关推荐

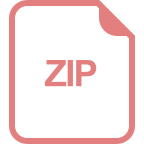
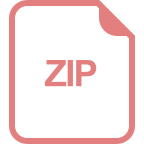
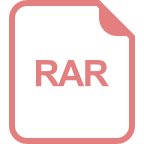

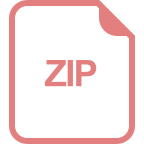
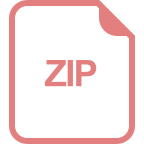
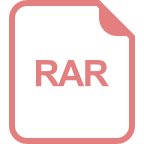
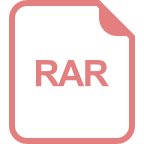
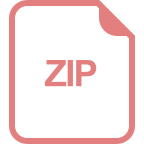
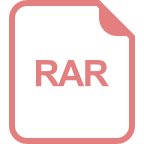
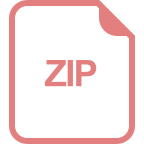
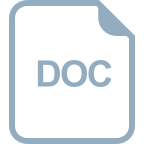
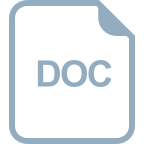

