如何计算一个二维向量(vector<vector<Point>>)每一个vector<Point>的面积,并打印出来?
时间: 2024-12-17 15:33:43 浏览: 1
在C++中,计算二维向量(`vector<vector<Point>>`)中的每个元素即`Point`类型的点的面积通常涉及到创建一个矩形区域,其边长由两个点决定。但是,除非我们有一个特殊的`Point`类定义了它的`x`和`y`坐标,否则直接计算点的面积是没有意义的,因为点没有大小。
假设你有一个包含`(x, y)`坐标的`Point`类,你可以这样做:
```cpp
#include <iostream>
#include <vector>
class Point {
public:
double x;
double y;
// 构造函数
Point(double x = 0, double y = 0) : x(x), y(y) {}
// 计算点到原点的距离(半径),这里我们假定点的面积就是半径的平方
double area() const { return x * x + y * y; }
};
// 遍历二维向量并打印每个点的面积
void printAreas(const std::vector<std::vector<Point>>& points) {
for (const auto& row : points) {
for (const auto& point : row) {
std::cout << "Area of point (" << point.x << ", " << point.y << "): " << point.area() << "\n";
}
std::cout << "---------\n"; // 每行结束时添加分隔线
}
}
int main() {
std::vector<std::vector<Point>> vec{{{1, 2}, {3, 4}}, {{5, 6}, {7, 8}}};
printAreas(vec);
return 0;
}
```
在这个例子中,我们首先定义了一个简单的`Point`类,它有一个成员函数`area()`,用于返回点到原点距离的平方。然后我们在`printAreas`函数中遍历整个二维向量,并对每个`Point`调用`area()`方法打印出它们的面积。
阅读全文
相关推荐
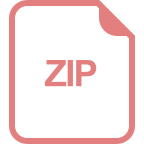
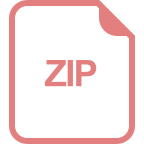
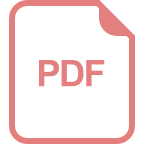















