vector<vector<cv::Point>>hull(contours0.size());
时间: 2024-05-20 17:11:36 浏览: 14
这行代码定义了一个名为"hull"的二维向量,其中包含了contours0向量的大小数量的元素。每个元素都是一个cv::Point类型的向量,表示的是对应轮廓的凸包点的坐标。
cv::Point是OpenCV库中用于表示二维点坐标的数据类型,该类型包含了两个成员变量x和y,分别表示点的横坐标和纵坐标。而contours0则是一个包含多个轮廓的向量,每个轮廓都是由一些点坐标组成的向量。因此,hull向量的大小就等于contours0向量的大小,每个元素都是一个cv::Point类型的向量,表示的是对应轮廓的凸包点的坐标。
相关问题
bool isPolygonInside(const std::vector<cv::Point>& polygon1, const std::vector<cv::Point>& polygon2, double& outsideArea) { // Check if all vertices of polygon1 are inside polygon2 bool allInside = true; for (const auto& vertex : polygon1) { double distance = cv::pointPolygonTest(polygon2, vertex, true); if (distance < 0) { allInside = false; break; } } if (allInside) { return true; } // Polygon1 is partially or completely outside polygon2 std::vector<std::vector<cv::Point>> intersectionPolygon; // 修改此处 if (cv::isContourConvex(polygon1) && cv::isContourConvex(polygon2)) { cv::Mat intersectionMat; cv::intersectConvexConvex(cv::Mat(polygon1), cv::Mat(polygon2), intersectionMat); if (cv::countNonZero(intersectionMat) > 0) { cv::findContours(intersectionMat, intersectionPolygon, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE); } } else { std::vector<cv::Point> hull1, hull2; cv::convexHull(polygon1, hull1); cv::convexHull(polygon2, hull2); std::vector<cv::Point> hullIntersection; cv::convexHull(hull1, hullIntersection, false, false); cv::fillConvexPoly(cv::Mat(hullIntersection), hull2, cv::Scalar(0), false); cv::findContours(cv::Mat(hullIntersection), intersectionPolygon, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE); } if (intersectionPolygon.empty()) { outsideArea = 0; return false; } double intersectionArea = std::abs(cv::contourArea(intersectionPolygon[0])); // 修改此处 double polygon1Area = std::abs(cv::contourArea(polygon1)); outsideArea = polygon1Area - intersectionArea; return true; // 修改此处 } 这个是你给的代码,运行后提示报错:terminate called after throwing an instance of 'cv::Exception' what(): OpenCV(4.5.0) /home/sniper/Downloads/opencv-4.5.0/modules/imgproc/src/contours.cpp:195: error: (-210:Unsupported format or combination of formats) [Start]FindContours supports only CV_8UC1 images when mode != CV_RETR_FLOODFILL otherwise supports CV_32SC1 images only in function 'cvStartFindContours_Impl'
根据报错信息,问题可能出现在 `cv::findContours()` 函数中。这个函数要求输入的图像是 CV_8UC1 格式的,但是你传递给函数的参数类型可能不正确。请检查你传递给 `cv::findContours()` 函数的参数类型是否正确。如果你的图像不是 CV_8UC1 格式,可以尝试将它转换为正确的格式。如果你仍然无法解决问题,请提供更多的代码和上下文信息,以便我可以更好地帮助你解决这个问题。
但是运行后报错:terminate called after throwing an instance of 'cv::Exception' what(): OpenCV(4.5.0) /home/sniper/Downloads/opencv-4.5.0/modules/imgproc/src/contours.cpp:1799: error: (-215:Assertion failed) (_contours.kind() == _InputArray::STD_VECTOR_VECTOR || _contours.kind() == _InputArray::STD_VECTOR_MAT || _contours.kind() == _InputArray::STD_VECTOR_UMAT) in function 'findContours'
这个错误通常是由于传递给 `findContours()` 函数的轮廓参数类型不正确导致的。
在你的代码中,`findContours()` 函数的第一个参数是一个 `cv::Mat` 类型的矩阵,而第二个参数 `intersectionPolygon` 是一个 `std::vector<cv::Point>` 类型的向量。这里的问题是,`findContours()` 函数期望的第二个参数是一个用于存储轮廓的输出向量,而你传递了一个点的向量。因此,你需要将 `intersectionPolygon` 声明为一个 `std::vector<std::vector<cv::Point>>` 类型的向量,以存储轮廓。
更改后的代码如下所示:
```
bool isPolygonInside(const std::vector<cv::Point>& polygon1, const std::vector<cv::Point>& polygon2, double& outsideArea) {
// Check if all vertices of polygon1 are inside polygon2
bool allInside = true;
for (const auto& vertex : polygon1) {
double distance = cv::pointPolygonTest(polygon2, vertex, true);
if (distance < 0) {
allInside = false;
break;
}
}
if (allInside) {
return true;
}
// Polygon1 is partially or completely outside polygon2
std::vector<std::vector<cv::Point>> intersectionPolygon; // 修改此处
if (cv::isContourConvex(polygon1) && cv::isContourConvex(polygon2)) {
cv::Mat intersectionMat;
cv::intersectConvexConvex(cv::Mat(polygon1), cv::Mat(polygon2), intersectionMat);
if (cv::countNonZero(intersectionMat) > 0) {
cv::findContours(intersectionMat, intersectionPolygon, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
}
} else {
std::vector<cv::Point> hull1, hull2;
cv::convexHull(polygon1, hull1);
cv::convexHull(polygon2, hull2);
std::vector<cv::Point> hullIntersection;
cv::convexHull(hull1, hullIntersection, false, false);
cv::fillConvexPoly(cv::Mat(hullIntersection), hull2, cv::Scalar(0), false);
cv::findContours(cv::Mat(hullIntersection), intersectionPolygon, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
}
if (intersectionPolygon.empty()) {
outsideArea = 0;
return false;
}
double intersectionArea = std::abs(cv::contourArea(intersectionPolygon[0])); // 修改此处
double polygon1Area = std::abs(cv::contourArea(polygon1));
outsideArea = polygon1Area - intersectionArea;
return true; // 修改此处
}
```
你需要将 `intersectionPolygon` 的类型从 `std::vector<cv::Point>` 改为 `std::vector<std::vector<cv::Point>>`,以及在计算交集面积时使用 `intersectionPolygon[0]` 来获取轮廓。
另外,最后一行需要返回 true,因为在计算出多边形1在多边形2外部的面积后,函数应该返回 true。
相关推荐
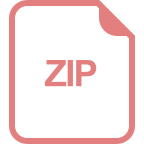
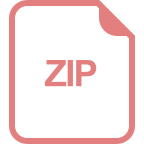
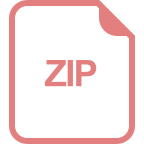








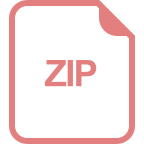
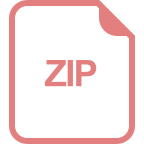
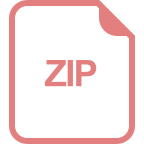
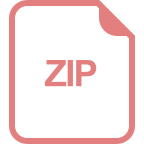