Qt 蓝牙主动读取 示例代码
时间: 2024-12-23 21:26:46 浏览: 5
Qt 提供了一套强大的蓝牙库,用于处理蓝牙功能。以下是使用Qt5的一个简单示例代码片段,展示了如何在QBluetoothDeviceDiscoveryAgent的帮助下,实现蓝牙设备的主动扫描并获取发现的设备列表:
```cpp
#include <QObject>
#include <QBluetooth>
#include <QBluetoothDeviceInfo>
class BluetoothScanner : public QObject
{
Q_OBJECT
public:
explicit BluetoothScanner(QObject *parent = nullptr)
: QObject(parent), discoveryAgent(new QBluetoothDeviceDiscoveryAgent(this))
{
// 设置代理并开始扫描
connect(discoveryAgent, &QBluetoothDeviceDiscoveryAgent::discoveredDevices, this, &BluetoothScanner::onDiscoveredDevices);
startScanning();
}
~BluetoothScanner()
{
stopScanning();
}
private slots:
void onDiscoveredDevices(const QSet<QBluetoothDeviceInfo>& devices)
{
foreach (const QBluetoothDeviceInfo &device, devices) {
qDebug() << "Found device: " << device.name() << ", Address: " << device.address().toString();
}
}
void startScanning()
{
if (!discoveryAgent->isScanning()) {
discoveryAgent->start();
}
}
void stopScanning()
{
if (discoveryAgent->isScanning()) {
discoveryAgent->stop();
}
}
signals:
void scanningFinished();
private:
QBluetoothDeviceDiscoveryAgent* discoveryAgent;
};
// 使用示例
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
BluetoothScanner scanner;
scanner.scanningFinished.connect([]{ qDebug() << "Scanning finished"; });
return app.exec();
}
```
在这个例子中,`BluetoothScanner` 类初始化了一个 `QBluetoothDeviceDiscoveryAgent` 实例,并连接到它的 `discoveredDevices` 信号,以便每当有新的蓝牙设备被发现时,会显示设备名称和地址。通过调用 `startScanning()` 开始扫描,`stopScanning()` 则停止扫描。
阅读全文
相关推荐
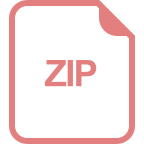
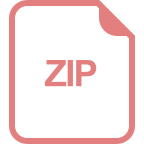
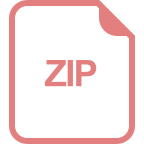


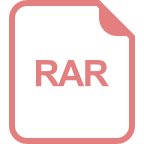
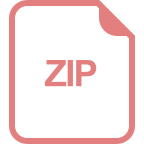
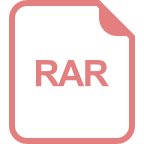
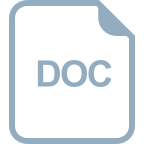
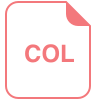
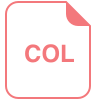






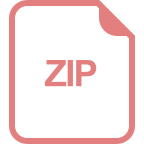
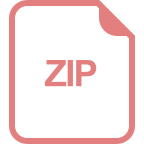