c语音编写程序,将摄氏温度(C)转换为华氏温度(F),转换公式为F=9C/5+36
时间: 2024-09-13 11:17:04 浏览: 18
要使用C语言编写程序,将摄氏温度转换为华氏温度,你可以按照以下步骤进行:
1. 首先,声明两个变量,一个用于存储摄氏温度的值,另一个用于存储转换后的华氏温度值。
2. 然后,提示用户输入摄氏温度的值。
3. 使用转换公式F=9*C/5+32计算华氏温度,将结果存储在之前声明的华氏温度变量中。
4. 最后,输出转换后的华氏温度值。
下面是一个简单的C语言程序示例代码:
```c
#include <stdio.h>
int main() {
float celsius, fahrenheit;
// 提示用户输入摄氏温度
printf("请输入摄氏温度: ");
scanf("%f", &celsius);
// 转换公式
fahrenheit = 9 * celsius / 5 + 32;
// 输出华氏温度
printf("华氏温度为: %.2f\n", fahrenheit);
return 0;
}
```
在这个程序中,`%f` 是格式化字符串,用于指示 `scanf` 和 `printf` 函数处理浮点数。`%.2f` 指示 `printf` 函数输出浮点数,并保留两位小数。
相关问题
编写程序,将摄氏温度(C)转换为华氏温度(F),转换公式为F=9C/5+36
要编写一个程序将摄氏温度转换为华氏温度,你需要根据给定的转换公式F = 9C/5 + 32来计算。这里是一个简单的示例,展示了如何在多种编程语言中实现这一功能:
1. 在Python中,可以这样实现:
```python
def celsius_to_fahrenheit(celsius):
fahrenheit = (9.0 / 5.0) * celsius + 32
return fahrenheit
# 使用函数
celsius_temp = float(input("请输入摄氏温度: "))
fahrenheit_temp = celsius_to_fahrenheit(celsius_temp)
print(f"摄氏温度 {celsius_temp}°C 等于华氏温度 {fahrenheit_temp}°F")
```
2. 在Java中,可以这样实现:
```java
public class TemperatureConverter {
public static void main(String[] args) {
double celsius = 0.0;
System.out.print("请输入摄氏温度: ");
celsius = Double.parseDouble(System.console().readLine());
double fahrenheit = celsiusToFahrenheit(celsius);
System.out.println("摄氏温度 " + celsius + "°C 等于华氏温度 " + fahrenheit + "°F");
}
public static double celsiusToFahrenheit(double celsius) {
return (9.0 / 5.0) * celsius + 32;
}
}
```
3. 在C++中,可以这样实现:
```cpp
#include <iostream>
using namespace std;
double celsiusToFahrenheit(double celsius) {
return (9.0 / 5.0) * celsius + 32;
}
int main() {
double celsius;
cout << "请输入摄氏温度: ";
cin >> celsius;
double fahrenheit = celsiusToFahrenheit(celsius);
cout << "摄氏温度 " << celsius << "°C 等于华氏温度 " << fahrenheit << "°F" << endl;
return 0;
}
```
这些代码示例都遵循了一个基本的逻辑流程:接收用户输入的摄氏温度值,使用转换公式计算出相应的华氏温度值,然后输出结果。
编写程序,讲摄氏度℃转为华氏温度F,转换公式位F=9C/5+32
以下是将摄氏度℃转为华氏温度F的程序:
```python
celsius = float(input("请输入摄氏度℃:"))
fahrenheit = 9 * celsius / 5 + 32
print("华氏温度为:", fahrenheit, "F")
```
如果您需要将其转换为 Lua 代码,可以使用以下代码:
```lua
io.write("请输入摄氏度℃:")
celsius = io.read("*number")
fahrenheit = 9 * celsius / 5 + 32
print("华氏温度为:", fahrenheit, "F")
```
对于“lua closure factory 完整代码”的问题,以下是一个示例:
```lua
function createCounter()
local count = 0
return function()
count = count + 1
return count
end
end
counter1 = createCounter()
print(counter1()) -- 输出 1
print(counter1()) -- 输出 2
counter2 = createCounter()
print(counter2()) -- 输出 1
print(counter1()) -- 输出 3
```
这是一个闭包工厂函数,它返回一个函数,该函数可以计算调用次数并返回计数器的当前值。每次调用 createCounter() 都会创建一个新的计数器,因此 counter1 和 counter2 是相互独立的。
对于“中文加密”的问题,这可能是一个比较广泛的问题,因此我需要更多的信息才能回答。如果您可以提供更多的上下文和详细信息,我将尽力回答您的问题。
相关推荐
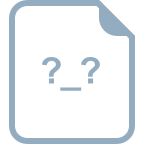
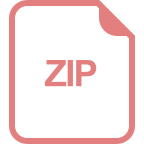
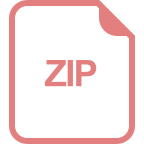












