假设以带头结点的循环链表表示队列,并且只设一个指针指向队尾元素节点(注意:不设头指针),试编写相应的置空队列,判断队列是否为空,入队和出队等算法
时间: 2024-12-15 08:19:19 浏览: 18
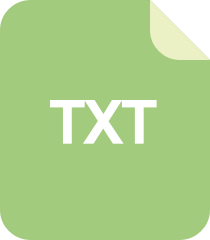
假设以带头结点的循环链表表示一个队列,并且只设一个队尾指针指向尾元素结点(注意不设头指针),试写出相应的置空队、入队、出队的算法 (Java)
在以单指针表示循环链表的队列结构中,由于只有一个指针`tail`指向队尾元素,我们可以这样实现队列的操作:
### 置空队列(Empty the Queue)
1. 设置`tail`为`NULL`,表示链表为空。
```c
void queue_empty(struct Node* &tail) {
tail = NULL;
}
```
### 判断队列是否为空(Is the Queue Empty?)
1. 检查`tail`是否为`NULL`,如果是,则队列为空。
```c
bool is_queue_empty(const struct Node* const tail) {
return tail == NULL;
}
```
### 入队(Enqueue)
1. 需要在队尾添加一个新节点,先创建一个新节点`new_node`。
2. 如果队列非空,将当前`tail`的`next`指向`new_node`。
3. 更新`tail`指向`new_node`,形成循环。
```c
void enqueue(struct Node** tail, char value) {
if (*tail) {
struct Node *new_node = new Node(value);
new_node->next = *tail;
*tail = new_node;
} else {
// 如果队列首次为空,创建一个单独的节点并设置其next为自身
struct Node *new_node = new Node(value);
*tail = new_node;
*tail->next = new_node;
}
}
```
### 出队(Dequeue)
1. 如果队列为空,返回错误或特殊值表示无法出队。
2. 否则,获取`tail`的下一个节点(即队首元素)为新的`tail`,然后删除该节点。
```c
char dequeue(struct Node** tail) {
if (!(*tail)) {
// 队列为空,处理异常情况
return -1; // 或者抛出异常
}
char value = (*tail)->data;
struct Node *temp = *tail;
*tail = (*tail)->next;
delete temp; // 删除节点
return value;
}
```
以上就是在这种队列结构下,实现置空、判断空、入队和出队的基本操作。
阅读全文
相关推荐
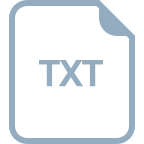
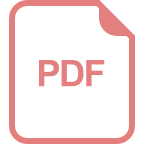












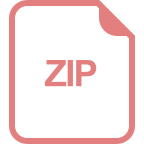