<template slot="htmlSlot" slot-scope="text"> <div v-html="text"></div> </template>转化vue3.x
时间: 2024-03-14 21:40:09 浏览: 14
在Vue 3.x中,`<template>`标签的`slot`属性已经被移除,取而代之的是使用`<slot>`标签来定义插槽内容。所以,你可以将原来的代码转化为以下形式:
```html
<template #htmlSlot="{ text }">
<div v-html="text"></div>
</template>
```
这样就可以在Vue 3.x中使用插槽了。
相关问题
需要<template slot-scope="scope">的值scope.row但是需要是<template slot="header">表头
在Vue.js中,使用`<template slot-scope="scope">`可以访问父组件传递给子组件的数据。而`scope.row`是指在表格中每一行的数据对象。当需要在表头中使用`scope.row`时,可以通过以下方式实现:
1. 在表格组件中定义一个`slot`,并将`scope.row`作为参数传递给子组件:
```html
<template slot="header" slot-scope="scope">
<!-- 在表头中使用scope.row -->
<th>{{ scope.row }}</th>
</template>
```
2. 在父组件中使用表格组件,并传递数据给子组件:
```html
<template>
<div>
<table-component>
<!-- 将scope.row作为参数传递给子组件 -->
<template slot="header" slot-scope="scope">
<th>{{ scope.row }}</th>
</template>
</table-component>
</div>
</template>
```
这样,你就可以在表头中使用`scope.row`的值了。
<template slot-scope="scope">的作用是什么
在Vue.js中,`<template slot-scope="scope">`是用于定义插槽作用域的语法。它的作用是允许父组件向子组件传递数据,并在子组件中使用这些数据进行渲染。
具体来说,当父组件中使用了`<slot>`标签,并且希望向插槽中传递数据时,可以使用`<template slot-scope="scope">`来定义插槽的作用域。通过`scope`对象,可以访问父组件传递的数据,并在子组件中进行处理和展示。
例如,假设有一个父组件传递了一个名为`user`的对象到子组件的插槽中,可以使用以下方式在子组件中接收和使用这个对象:
```html
<template slot-scope="scope">
<div>{{ scope.user.name }}</div>
<div>{{ scope.user.age }}</div>
</template>
```
在上述代码中,`scope`对象就是父组件传递的数据对象,可以通过`scope.user.name`和`scope.user.age`来访问其中的属性。
相关推荐
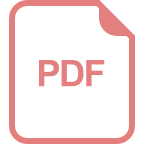
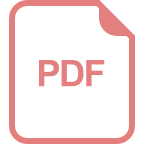
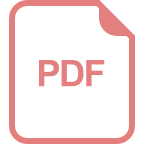












