功能需求:复制文件 •输入:./copy copy.c new.c •描述:复制一份copy的文件并重新命名为new • 要求:只能用open/read/write/close函数 •形式:代码/伪代码/流程图,任一即可
时间: 2024-09-11 11:16:28 浏览: 104
这是一个简单的命令行脚本的伪代码示例,用于说明如何使用`open`, `read`, 和 `write` 函数来复制文件。假设我们是在Unix/Linux环境中:
```cpp
// 伪代码示例
#include <stdio.h> // 使用标准I/O库,包含open, read, write, close
void copy_file(const char* src_filename, const char* dst_filename) {
// 打开源文件进行读取
int src_fd = open(src_filename, O_RDONLY); // 使用只读模式打开
if (src_fd == -1) {
perror("Failed to open source file");
return;
}
// 创建或打开目标文件进行写入
int dst_fd = open(dst_filename, O_WRONLY | O_CREAT | O_TRUNC, S_IRUSR | S_IWUSR);
if (dst_fd == -1) {
perror("Failed to create target file");
close(src_fd); // 关闭源文件
return;
}
// 读取源文件内容,并写入目标文件
off_t bytes_read;
char buffer[4096]; // 假设每次读取4K字节
while ((bytes_read = read(src_fd, buffer, sizeof(buffer))) > 0) {
write(dst_fd, buffer, bytes_read);
}
// 如果读取失败,则报告错误并关闭文件
if (bytes_read == -1) {
perror("Error reading from source file");
close(src_fd);
close(dst_fd);
return;
}
// 成功后关闭所有文件
close(src_fd);
close(dst_fd);
printf("File copied successfully.\n");
}
int main() {
copy_file("./copy.c", "new.c");
return 0;
}
```
这个伪代码展示了基本的步骤:打开源文件,创建或打开目标文件,然后逐块读取源文件的内容并写入目标文件。请注意,实际操作中需要处理可能出现的异常。
阅读全文
相关推荐
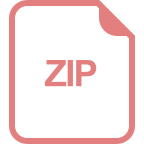
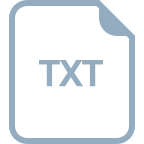
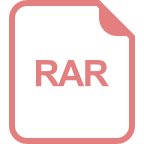









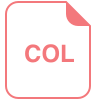



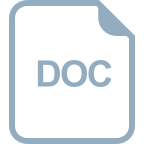
