如何利用Servlet技术实现一个购物车功能,并使用Map存储购物车中的书籍对象?请提供一个具体的实现示例。
时间: 2024-10-30 08:16:39 浏览: 17
在Web应用开发中,实现购物车功能通常涉及到会话管理、商品对象设计以及会话中数据的存储和操作。根据你提供的关键词,这里会介绍如何在Servlet中实现一个购物车功能,以存储书籍对象和用户选择的书籍数量。假设我们已经有了`BookBean`类和`CartItemBean`类,我们将通过`HttpSession`来跟踪用户的购物车状态。
参考资源链接:[Servlet实现购物车:添加、查看书籍](https://wenku.csdn.net/doc/fm7k0fhop4?spm=1055.2569.3001.10343)
首先,我们需要在Servlet中创建一个会话对象,如果会话不存在,`request.getSession()`将会创建一个新的会话:
```java
HttpSession session = request.getSession(true);
```
接着,我们可以获取会话中存储购物车的`Map`对象,如果不存在,则初始化一个新的`HashMap`:
```java
Map<String, CartItemBean> cartMap = (Map<String, CartItemBean>) session.getAttribute(
参考资源链接:[Servlet实现购物车:添加、查看书籍](https://wenku.csdn.net/doc/fm7k0fhop4?spm=1055.2569.3001.10343)
相关问题
如何在Servlet环境中通过Map存储购物车中的书籍对象,并实现添加、修改书籍数量以及查看购物车内容的功能?
在Servlet中实现购物车功能,首先需要了解如何利用会话(session)来跟踪用户的状态。会话管理允许我们在用户的浏览器和服务器之间共享信息,这对于实现购物车至关重要。在Servlet API中,可以使用`request.getSession()`方法来获取当前用户的会话对象,并通过`session.setAttribute()`方法存储购物车对象。购物车对象通常是`Map`类型的,其中键(Key)是书籍的唯一标识(如ISBN),值(Value)是`CartItemBean`对象,这个对象包含了商品信息和购买数量。
参考资源链接:[Servlet实现购物车:添加、查看书籍](https://wenku.csdn.net/doc/fm7k0fhop4?spm=1055.2569.3001.10343)
下面是实现添加书籍到购物车的具体步骤和示例代码:
1. 创建`CartItemBean`类,包含以下属性和方法:
```java
public class CartItemBean {
private BookBean book;
private int quantity;
public CartItemBean(BookBean book, int quantity) {
this.book = book;
this.quantity = quantity;
}
// Getter和Setter方法
// ...
}
```
2. 在Servlet中处理添加书籍到购物车的请求:
```java
HttpSession session = request.getSession();
Map<String, CartItemBean> cart = (Map<String, CartItemBean>) session.getAttribute(
参考资源链接:[Servlet实现购物车:添加、查看书籍](https://wenku.csdn.net/doc/fm7k0fhop4?spm=1055.2569.3001.10343)
那么请给我一个只用Servlet和JSP实现的购物车例子,不要用到Java Scrip
好的,这里给您提供一个简单的购物车例子,只使用Servlet和JSP实现。
首先,在购物车中需要实现添加商品、删除商品和修改商品数量的功能。我们可以通过在Session中保存购物车的信息来实现这些功能。
在添加商品时,我们可以在Servlet中获取商品的信息,将商品信息保存在一个JavaBean中,并将JavaBean对象添加到Session中。在JSP中,我们可以通过JSTL标签库来遍历Session中保存的JavaBean对象,将购物车中的商品展示出来。
在删除商品时,我们需要获取要删除的商品的信息,然后遍历Session中保存的JavaBean对象,找到对应的商品并将其从Session中移除。在JSP中,我们可以通过JSTL标签库来重新展示购物车中的商品列表。
在修改商品数量时,我们可以通过在JSP中使用表单来获取用户输入的数量,并将数量信息保存在Session中。在展示购物车中的商品列表时,我们可以根据Session中保存的数量信息来展示对应商品的数量,并在用户修改数量时更新Session中保存的数量信息。
这里是一个简单的购物车示例代码,您可以参考一下:
CartServlet.java:
```java
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("/cart")
public class CartServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String action = request.getParameter("action");
HttpSession session = request.getSession();
Cart cart = (Cart) session.getAttribute("cart");
if (cart == null) {
cart = new Cart();
session.setAttribute("cart", cart);
}
if ("add".equals(action)) {
int id = Integer.parseInt(request.getParameter("id"));
String name = request.getParameter("name");
double price = Double.parseDouble(request.getParameter("price"));
int quantity = Integer.parseInt(request.getParameter("quantity"));
Product product = new Product(id, name, price);
cart.addItem(product, quantity);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
} else if ("delete".equals(action)) {
int id = Integer.parseInt(request.getParameter("id"));
Product product = new Product(id, "", 0);
cart.removeItem(product);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
} else if ("update".equals(action)) {
List<CartItem> items = new ArrayList<CartItem>();
for (Product product : cart.getItems().keySet()) {
int quantity = Integer.parseInt(request.getParameter("quantity_" + product.getId()));
items.add(new CartItem(product, quantity));
}
cart.updateItems(items);
response.sendRedirect(request.getContextPath() + "/cart.jsp");
} else {
response.sendRedirect(request.getContextPath() + "/cart.jsp");
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request, response);
}
}
```
Cart.java:
```java
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Cart {
private Map<Product, Integer> items;
public Cart() {
items = new HashMap<Product, Integer>();
}
public void addItem(Product product, int quantity) {
int newQuantity = quantity;
if (items.containsKey(product)) {
newQuantity += items.get(product);
}
items.put(product, newQuantity);
}
public void removeItem(Product product) {
items.remove(product);
}
public Map<Product, Integer> getItems() {
return items;
}
public double getTotal() {
double total = 0;
for (Product product : items.keySet()) {
total += product.getPrice() * items.get(product);
}
return total;
}
public void updateItems(List<CartItem> cartItems) {
for (CartItem cartItem : cartItems) {
Product product = cartItem.getProduct();
int quantity = cartItem.getQuantity();
if (quantity <= 0) {
items.remove(product);
} else {
items.put(product, quantity);
}
}
}
}
```
CartItem.java:
```java
public class CartItem {
private Product product;
private int quantity;
public CartItem(Product product, int quantity) {
this.product = product;
this.quantity = quantity;
}
public Product getProduct() {
return product;
}
public int getQuantity() {
return quantity;
}
}
```
Product.java:
```java
public class Product {
private int id;
private String name;
private double price;
public Product(int id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
}
```
cart.jsp:
```jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>购物车</title>
</head>
<body>
<h1>购物车</h1>
<table border="1">
<tr>
<th>ID</th>
<th>名称</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
<c:forEach items="${cart.items}" var="entry">
<tr>
<td>${entry.key.id}</td>
<td>${entry.key.name}</td>
<td>${entry.key.price}</td>
<td>
<form method="post" action="${pageContext.request.contextPath}/cart">
<input type="hidden" name="action" value="update">
<input type="hidden" name="id" value="${entry.key.id}">
<input type="text" name="quantity_${entry.key.id}" value="${entry.value}">
<input type="submit" value="更新">
</form>
</td>
<td>${entry.key.price * entry.value}</td>
<td>
<form method="post" action="${pageContext.request.contextPath}/cart">
<input type="hidden" name="action" value="delete">
<input type="hidden" name="id" value="${entry.key.id}">
<input type="submit" value="删除">
</form>
</td>
</tr>
</c:forEach>
<tr>
<td colspan="4">总计:</td>
<td>${cart.total}</td>
<td></td>
</tr>
</table>
<h2>添加商品</h2>
<form method="post" action="${pageContext.request.contextPath}/cart">
<input type="hidden" name="action" value="add">
ID:<input type="text" name="id"><br>
名称:<input type="text" name="name"><br>
单价:<input type="text" name="price"><br>
数量:<input type="text" name="quantity"><br>
<input type="submit" value="添加">
</form>
</body>
</html>
```
在这个例子中,我们创建了一个Cart类来保存购物车的信息,其中包括了添加商品、删除商品和修改商品数量的方法。在Servlet中,我们根据用户的操作来调用Cart类的相应方法,并将修改后的购物车信息保存在Session中。在JSP中,我们使用JSTL标签库来遍历Session中保存的购物车信息,并展示购物车中的商品列表。同时,我们还使用了表单来获取用户输入的信息,并将其传递给Servlet来完成添加商品的操作。
这个例子比较简单,仅供参考。在实际开发中,还需要考虑数据校验、安全性等问题。
阅读全文
相关推荐
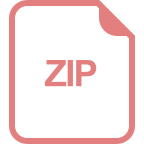
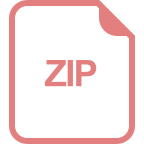
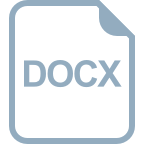
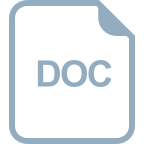
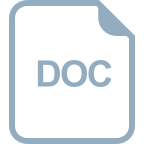
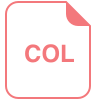








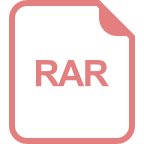
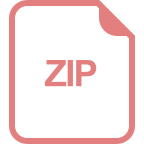