java netty发送邮件
时间: 2023-07-28 22:08:52 浏览: 208
Netty是一个网络编程框架,它主要用于构建高性能、高可靠性的网络应用程序。然而,Netty本身并不提供发送邮件的功能,它更适用于实现基于TCP或UDP的网络通信。
如果你想在Java中使用Netty发送邮件,你可以借助JavaMail API来实现。JavaMail API是Java平台的标准API,它提供了发送和接收电子邮件的功能。
以下是一个使用Netty和JavaMail API发送简单邮件的示例代码:
```java
import io.netty.util.concurrent.Future;
import io.netty.util.concurrent.FutureListener;
import io.netty.util.concurrent.Promise;
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import javax.mail.internet.MimeBodyPart;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import java.util.Properties;
public class EmailSender {
public static void main(String[] args) {
// 配置SMTP服务器和认证信息
Properties props = new Properties();
props.put("mail.smtp.host", "smtp.example.com");
props.put("mail.smtp.port", "587");
props.put("mail.smtp.auth", "true");
// 创建会话
Session session = Session.getInstance(props, new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your_username", "your_password");
}
});
// 创建邮件消息
MimeMessage message = new MimeMessage(session);
try {
// 设置发件人
message.setFrom(new InternetAddress("sender@example.com"));
// 设置收件人
message.addRecipient(Message.RecipientType.TO, new InternetAddress("recipient@example.com"));
// 设置主题
message.setSubject("Test Email");
// 创建正文部分
MimeBodyPart textPart = new MimeBodyPart();
textPart.setText("This is the email content.");
// 创建附件部分
MimeBodyPart attachmentPart = new MimeBodyPart();
FileDataSource fileDataSource = new FileDataSource("path_to_attachment_file");
attachmentPart.setDataHandler(new DataHandler(fileDataSource));
attachmentPart.setFileName(fileDataSource.getName());
// 创建多部分消息
MimeMultipart multipart = new MimeMultipart();
multipart.addBodyPart(textPart);
multipart.addBodyPart(attachmentPart);
// 设置消息内容
message.setContent(multipart);
// 使用Netty发送邮件
Promise<Void> promise = new DefaultPromise<>(session.channel().eventLoop());
Transport transport = session.getTransport("smtp");
transport.connect();
transport.sendMessage(message, message.getAllRecipients(), new SendListener(promise));
transport.close();
// 等待邮件发送完成
Future<Void> future = promise;
future.addListener(new FutureListener<Void>() {
@Override
public void operationComplete(Future<Void> future) throws Exception {
if (future.isSuccess()) {
System.out.println("Email sent successfully.");
} else {
System.out.println("Failed to send email: " + future.cause().getMessage());
}
}
});
} catch (Exception ex) {
ex.printStackTrace();
}
}
private static class SendListener extends SendMailTransportListener {
private final Promise<Void> promise;
public SendListener(Promise<Void> promise) {
this.promise = promise;
}
@Override
public void messageDelivered(TransportEvent e) {
promise.setSuccess(null);
}
@Override
public void messageNotDelivered(TransportEvent e) {
promise.setFailure(e.getException());
}
@Override
public void messagePartiallyDelivered(TransportEvent e) {
promise.setFailure(e.getException());
}
}
}
```
请注意,上述代码中的SMTP服务器和认证信息需要根据你的实际情况进行配置。另外,需要将"sender@example.com"和"recipient@example.com"替换为实际的发件人和收件人邮箱地址。最后,将"path_to_attachment_file"替换为要附加的文件的路径。
这只是一个简单的示例,你可以根据自己的需求进行扩展和定制。希望对你有帮助!
阅读全文
相关推荐
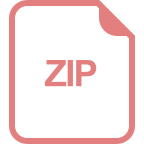
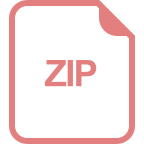
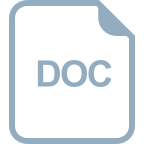
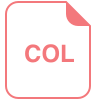
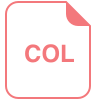
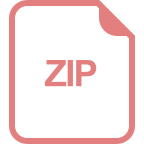
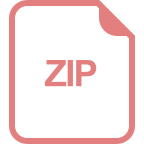
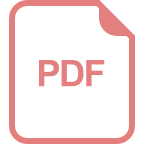
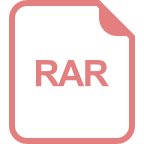
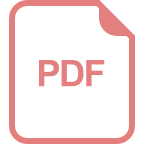
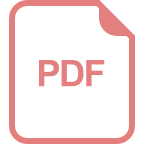
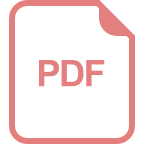
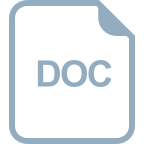
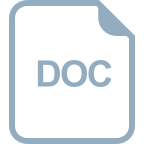
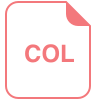