C#如何获取远程linux的某一个进程ID
时间: 2024-11-09 13:24:36 浏览: 9
在C#中,要从远程Linux服务器获取某个进程ID,可以使用`SSH.NET`库结合命令行工具(如`ps`)来查找进程。这里是一个基本步骤的例子:
```csharp
using SSH.NET;
public int GetRemoteProcessId(string serverIp, string username, string password, string processName)
{
int processId = 0; // 初始化结果
using (var client = new SshClient(serverIp))
{
// 设置SSH连接信息
client.ConnectionInfo.AuthenticationMethod = AuthenticationMethod.Password;
client.ConnectionInfo.Username = username;
client.ConnectionInfo.Password = password;
try
{
client.Connect();
// 创建shell通道,执行命令
using (var shell = client.CreateShell())
{
var command = $"ps aux | grep '{processName}'"; // 查询包含进程名的进程列表
var output = shell.Run(command);
// 搜索输出结果,寻找进程ID
foreach (var line in output.StandardOutput.Lines)
{
var parts = line.Split(' ');
if (parts.Length >= 3 && parts[0] == processName)
{
// 根据Unix系统格式提取进程ID(通常是第三个元素)
processId = int.Parse(parts[1]);
break;
}
}
// 如果找不到则返回0或抛异常
if (processId == 0)
{
throw new Exception($"无法找到进程 ID,名为'{processName}'的进程不存在或权限不足");
}
}
}
catch (Exception ex)
{
Console.WriteLine($"错误:{ex.Message}");
}
finally
{
client.Disconnect();
}
return processId;
}
}
```
这个函数会在远程服务器上执行`ps aux | grep <processName>`命令,然后遍历输出的行来找出匹配的进程ID。你需要将`processName`参数替换为你想要查找的实际进程名称。
阅读全文
相关推荐
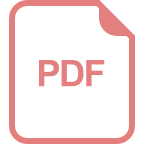
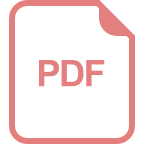
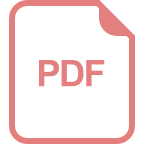




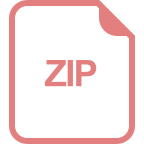
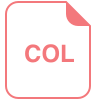
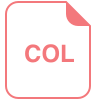
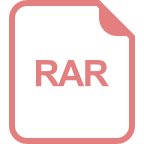
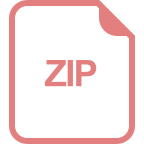
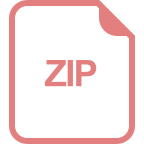
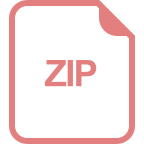
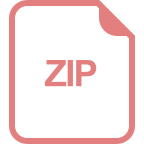
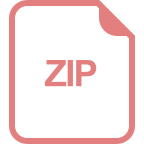