如何用C++编程实现计算阶乘的倒数并将其累加求和?
时间: 2024-10-21 13:14:01 浏览: 4
在C++中,你可以通过定义一个函数来计算阶乘的倒数,然后将所有阶乘的倒数累加求和。首先,你需要创建一个递归或循环方法来计算阶乘,然后计算其倒数。接着,你可以使用`std::accumulate`函数或者自定义一个累加过程来求和。
以下是一个简单的示例:
```cpp
#include <iostream>
#include <numeric> // 包含accumulate函数
// 计算阶乘
unsigned long long factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
// 计算阶乘的倒数
double reciprocal_factorial(int n) {
unsigned long long fact = factorial(n);
if (fact != 0)
return 1.0 / fact;
else // 防止分母为零的情况
return std::numeric_limits<double>::quiet_NaN(); // 返回非数字值
}
// 累加阶乘的倒数求和
double sum_reciprocals(int start, int end) {
double sum = 0;
for (int i = start; i <= end; ++i)
sum += reciprocal_factorial(i);
return sum;
}
int main() {
int range_start = 1;
int range_end = 5; // 指定计算范围
double result = sum_reciprocals(range_start, range_end);
std::cout << "The sum of reciprocals of factorials from " << range_start << " to " << range_end << " is: " << result << std::endl;
return 0;
}
```
在这个例子中,我们假设阶乘不会超过`unsigned long long`类型的限制,如果需要处理更大的数值,可以考虑使用高精度数学库,如GMP。
阅读全文
相关推荐
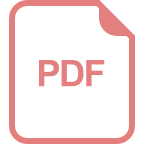
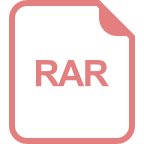
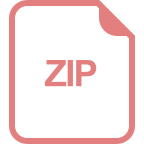
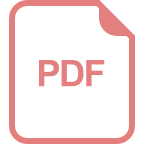
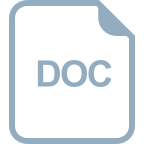
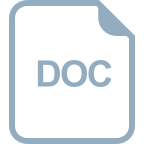
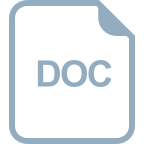
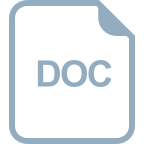
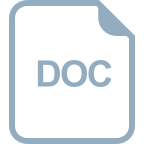

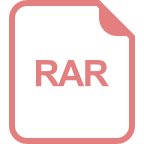
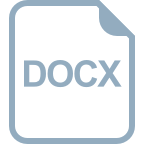