float_random = random.uniform(0, 1) 如何生成一个(1,10)大小的数组
时间: 2024-11-04 14:13:47 浏览: 26
如果你想生成一个介于1到10之间的浮点数数组,而不是单个值,你可以使用numpy库中的`arange`函数或`linspace`函数。以下是两种方法的示例:
1. 使用`numpy.arange`:
```python
import numpy as np
float_random_array = np.arange(1, 11).astype(float) / 10.0 # 将整数数组转换为浮点数,得到范围在0到1之间的小数
```
这里生成的是一个包含从1到10共10个等差值的数组,然后除以10将其缩放到指定范围内。
2. 使用`numpy.linspace`:
```python
float_random_array = np.linspace(start=1, stop=10, num=10, endpoint=True, dtype=float)
```
`linspace`函数会生成从start到stop的num个等间距的点,包括endpoint。同样,结果也是范围在0到1之间的浮点数。
这两种方法都会生成一个长度为10,数值范围在1到10(包含1和10)的浮点数数组。
相关问题
with tf.variable_scope(self.scope): self.dense1_mul = dense(self.state, dense1_size, weight_init=tf.random_uniform_initializer((-1/tf.sqrt(tf.to_float(self.state_dims))), 1/tf.sqrt(tf.to_float(self.state_dims))), bias_init=tf.random_uniform_initializer((-1/tf.sqrt(tf.to_float(self.state_dims))), 1/tf.sqrt(tf.to_float(self.state_dims))), scope='dense1') self.dense1 = relu(self.dense1_mul, scope='dense1') self.dense2_mul = dense(self.dense1, dense2_size, weight_init=tf.random_uniform_initializer((-1/tf.sqrt(tf.to_float(dense1_size))), 1/tf.sqrt(tf.to_float(dense1_size))), bias_init=tf.random_uniform_initializer((-1/tf.sqrt(tf.to_float(dense1_size))), 1/tf.sqrt(tf.to_float(dense1_size))), scope='dense2') self.dense2 = relu(self.dense2_mul, scope='dense2') self.output_mul = dense(self.dense2, self.action_dims, weight_init=tf.random_uniform_initializer(-1*final_layer_init, final_layer_init), bias_init=tf.random_uniform_initializer(-1*final_layer_init, final_layer_init), scope='output') self.output_tanh = tanh(self.output_mul, scope='output') # Scale tanh output to lower and upper action bounds self.output = tf.multiply(0.5, tf.multiply(self.output_tanh, (self.action_bound_high-self.action_bound_low)) + (self.action_bound_high+self.action_bound_low)) self.network_params = tf.trainable_variables(scope=self.scope) self.bn_params = []
这段代码是一个神经网络的定义,包含了三个全连接层。其中第一层的输入是状态(state),输出的大小为dense1_size。第二层的输入则是第一层的输出,输出的大小为dense2_size。最后一层的输入是第二层的输出,输出的大小是动作(action)的维度(action_dims)。每个全连接层都包含一个线性变换和一个非线性激活函数,其中第一层和第二层使用的激活函数为ReLU,最后一层使用的激活函数为tanh。该神经网络的输出会被缩放到动作的上下限(action_bound_high和action_bound_low)之间。所有的可训练变量都被存储在网络参数(network_params)中。
class myModel: def __init__(self): #################### '''声明模型对应的参数''' #################### self.W1 = tf.Variable(shape=[28 * 28, 100], dtype=tf.float32, # 定义w1 28*28 为input 784个点 100为中间层 initial_value=tf.random.uniform(shape=[28 * 28, 100], minval=-0.1, maxval=0.1)) # 最小值最大值设定为0.1 self.b1 = tf.Variable(shape=[100], dtype=tf.float32, initial_value=tf.zeros(100)) # 定义b1 self.W2 = tf.Variable(shape=[100, 10], dtype=tf.float32, # 定义W2 initial_value=tf.random.uniform(shape=[100, 10], # 输出为10个 minval=-0.1, maxval=0.1)) self.b2 = tf.Variable(shape=[10], dtype=tf.float32, initial_value=tf.zeros(10)) # 定义b2 self.trainable_variables = [self.W1, self.W2, self.b1, self.b2] # 输出w1,w2,b1,b2各个值,这段代码的含义是什么
这段代码定义了一个名为 "myModel" 的类,该类包含了一个初始化函数 "__init__" ,在该函数中定义了模型的参数。其中,W1、W2、b1、b2 分别表示模型的权重和偏差,是 Tensorflow 的 Variable 类型,代表可训练的参数。trainable_variables 列表包含了所有可训练的参数,用于在训练模型时更新这些参数的值。这段代码的作用是创建一个简单的全连接神经网络模型,并定义了模型的参数。
阅读全文
相关推荐
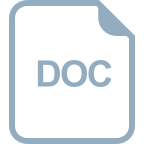
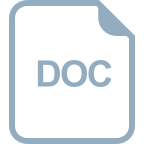
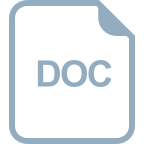














