实验1:用python设计一个Shape类,该类有一个属性name,并有一个方法 area(),该方法在子类中需要被重写。然后设计两个子类Circle和 Rectangle,分别计算圆形和矩形的面积。
时间: 2024-12-23 10:18:42 浏览: 7
实验1的设计可以按照以下步骤进行:
首先,我们创建一个名为`Shape`的基类,它有一个属性`name`表示形状名称,以及一个抽象方法`area()`:
```python
class Shape:
def __init__(self, name):
self.name = name
def area(self):
raise NotImplementedError("子类必须实现这个方法")
```
接着,我们为`Shape`类创建两个子类`Circle`和`Rectangle`,它们继承自`Shape`并实现了各自的`area()`方法:
```python
from math import pi
class Circle(Shape):
def __init__(self, radius):
super().__init__("Circle")
self.radius = radius
def area(self):
return pi * (self.radius ** 2)
class Rectangle(Shape):
def __init__(self, width, height):
super().__init__("Rectangle")
self.width = width
self.height = height
def area(self):
return self.width * self.height
```
现在,你可以实例化这两个子类并调用`area()`方法:
```python
circle = Circle(5)
print(circle.area()) # 输出:78.54
rectangle = Rectangle(10, 6)
print(rectangle.area()) # 输出:60
```
在这个例子中,`Shape`是一个抽象基类,而`Circle`和`Rectangle`是具体的实现类,通过重写了`area()`方法,展示了多态性。
阅读全文
相关推荐
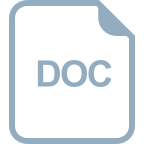
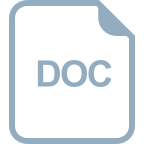
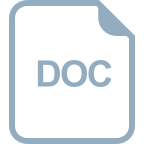













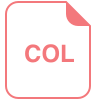


