for (auto itr = record.MemberBegin(); itr != record.MemberEnd(); ++itr)获取当前迭代器位置索引
时间: 2024-09-15 20:02:19 浏览: 67
在 C++ 中,如果你使用的是 STL 容器,比如 `std::vector` 或者 `std::list`,你可以通过迭代器来访问元素。迭代器本身并不直接提供索引的功能,但是在 C++11 之后,你可以使用 `std::distance` 函数来计算两个迭代器之间的距离,这在某种程度上可以用来获得迭代器的“索引”。
对于 `std::vector` 这样的连续存储容器,使用 `std::distance` 来获取当前迭代器位置相对于容器开始位置的索引是效率很高的,因为它只需要计算偏移量。而对于 `std::list` 或其他非连续存储的容器,这可能会导致线性时间的复杂度,因为需要遍历整个容器直到达到当前迭代器位置。
下面是如何使用 `std::distance` 来获取迭代器索引的例子:
```cpp
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto itr = vec.begin(); // 迭代器指向容器的第一个元素
auto index = std::distance(vec.begin(), itr); // 获取迭代器位置索引
std::cout << "The index of the current iterator position is: " << index << std::endl;
// 增加迭代器
++itr;
index = std::distance(vec.begin(), itr); // 更新索引值
std::cout << "After incrementing, the index is: " << index << std::endl;
return 0;
}
```
输出将会是:
```
The index of the current iterator position is: 0
After incrementing, the index is: 1
```
注意,这里的代码是基于你提供的迭代器是来自一个 `std::vector`。如果你的 `record` 对象不是使用 `std::vector` 或类似的容器实现的,那么上述方法可能不适用。
阅读全文
相关推荐
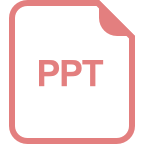
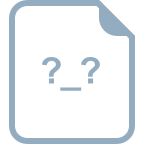
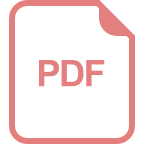















