用遗传算法对目标函数寻优的Python代码
时间: 2024-04-24 19:21:48 浏览: 16
当使用遗传算法来对目标函数进行优化时,您可以参考以下的Python代码示例:
```python
import random
import numpy as np
# 目标函数(示例:要寻找的最小值)
def objective_function(x):
return x**2
# 初始化种群
def initialize_population(population_size, chromosome_length, lower_bound, upper_bound):
population = []
for _ in range(population_size):
chromosome = [random.uniform(lower_bound, upper_bound) for _ in range(chromosome_length)]
population.append(chromosome)
return population
# 计算适应度值
def calculate_fitness(population):
fitness_values = []
for chromosome in population:
fitness = objective_function(chromosome[0])
fitness_values.append(fitness)
return fitness_values
# 选择操作(轮盘赌选择)
def selection(population, fitness_values):
probabilities = np.array(fitness_values) / sum(fitness_values)
selected_population = random.choices(population, probabilities, k=len(population))
return selected_population
# 交叉操作(单点交叉)
def crossover(parent1, parent2):
crossover_point = random.randint(0, len(parent1) - 1)
offspring = parent1[:crossover_point] + parent2[crossover_point:]
return offspring
# 变异操作(高斯变异)
def mutation(individual, mutation_rate, lower_bound, upper_bound):
mutated_individual = []
for gene in individual:
if random.random() < mutation_rate:
mutated_gene = gene + random.gauss(0, 1)
mutated_gene = max(min(mutated_gene, upper_bound), lower_bound)
mutated_individual.append(mutated_gene)
else:
mutated_individual.append(gene)
return mutated_individual
# 遗传优化算法主函数
def genetic_algorithm(population_size, chromosome_length, lower_bound, upper_bound, generations, mutation_rate):
population = initialize_population(population_size, chromosome_length, lower_bound, upper_bound)
for _ in range(generations):
fitness_values = calculate_fitness(population)
selected_population = selection(population, fitness_values)
new_population = []
while len(new_population) < population_size:
parent1 = random.choice(selected_population)
parent2 = random.choice(selected_population)
offspring = crossover(parent1, parent2)
offspring = mutation(offspring, mutation_rate, lower_bound, upper_bound)
new_population.append(offspring)
population = new_population
best_individual = min(population, key=lambda x: objective_function(x[0]))
return best_individual
# 示例用法
population_size = 100
chromosome_length = 1
lower_bound = -10
upper_bound = 10
generations = 50
mutation_rate = 0.1
best_individual = genetic_algorithm(population_size, chromosome_length, lower_bound, upper_bound, generations, mutation_rate)
print("Best Individual:", best_individual)
print("Best Fitness:", objective_function(best_individual[0]))
```
在这个示例代码中,我们假设目标函数是一个简单的函数 `x^2`。您可以根据实际情况修改目标函数的定义。遗传算法的其他操作,如选择操作、交叉操作和变异操作,也可以根据需求进行修改和扩展。
请注意,这个示例代码中的遗传算法是针对单变量优化问题的。如果您需要解决多变量优化问题,可以相应地修改染色体的长度和适应度计算方法。
希望这个示例对您有帮助!
相关推荐
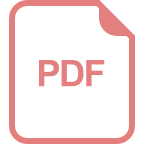
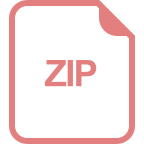
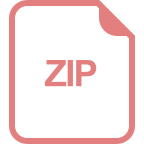














