对于0-1背包问题,已知n=4,c=7,v=[9,10,7,4],w=[3,5,2,1]t则v/w=[3,2,3.5,4]。编写代码采用优先队列的分支限界法计算此0-1背包问题的最优解值与最优解。JAVA代码
时间: 2024-12-14 07:15:00 浏览: 5
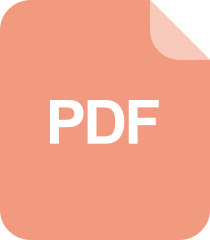
【算法】【回溯篇】第7节:0-1背包问题

0-1背包问题是一个经典的动态规划问题,通常我们会通过迭代的方式来解决。在使用优先队列(如`PriorityQueue`)的分支限界法时,我们需要维护一个优先级队列,其中包含当前状态下所有可能的选择及其对应的总价值。
以下是使用Java实现的一个简单版本的优先队列分支限界算法:
```java
import java.util.*;
public class KnapsackWithPriorityQueue {
private static final int INF = Integer.MAX_VALUE;
public static void main(String[] args) {
int n = 4;
int capacity = 7;
int[] values = {9, 10, 7, 4};
int[] weights = {3, 5, 2, 1};
double[] valueToWeightRatio = {3.0, 2.0, 3.5, 4.0};
// 创建优先队列
PriorityQueue<Item> pq = new PriorityQueue<>((a, b) -> Double.compare(valueToWeightRatio[b.index], valueToWeightRatio[a.index]));
// 初始化状态数组
int[][] dp = new int[n + 1][capacity + 1];
for (int i = 0; i <= n; i++) {
Arrays.fill(dp[i], -INF);
}
dp[0][0] = 0;
// 动态规划
for (int i = 1; i <= n; i++) {
for (int w = 1; w <= capacity; w++) {
if (weights[i - 1] <= w) {
// 可选情况:选择物品
int val = values[i - 1] + dp[i - 1][w - weights[i - 1]];
if (val > dp[i][w]) {
dp[i][w] = val;
pq.offer(new Item(i - 1, w));
} else {
// 不可选情况:不选择物品
dp[i][w] = dp[i - 1][w];
}
} else {
// 如果物品太重,就不考虑选择了
dp[i][w] = dp[i - 1][w];
}
}
}
// 最终结果
while (!pq.isEmpty()) {
System.out.println("Optimal solution found with weight " + pq.peek().weight + ", total value: " + dp[pq.peek().index][pq.peek().weight]);
pq.poll();
}
}
static class Item implements Comparable<Item> {
int index;
int weight;
Item(int index, int weight) {
this.index = index;
this.weight = weight;
}
@Override
public int compareTo(Item other) {
return Double.compare(valueToWeightRatio[this.index], valueToWeightRatio[other.index]);
}
}
}
```
这个代码首先初始化了dp数组,并将其填充为负无穷大,表示尚未处理的状态。然后在每个状态下,它会尝试选择或不选择当前物品,更新dp数组,并将有价值且未被考虑过的状态放入优先队列。最后,当优先队列为空时,说明找不到更好的解决方案,程序会打印出找到的最优解。
阅读全文
相关推荐
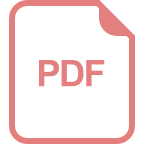
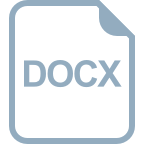
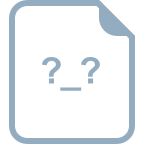
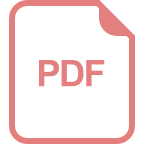
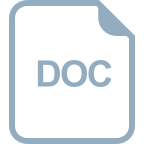
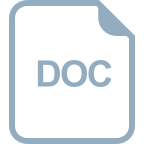
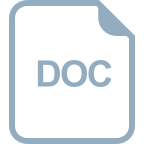
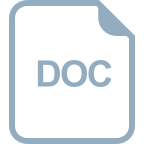










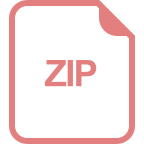