请用numpy 实现一个transformer 网络,网络包括自注意,5层encoder 和 6层decoder, 不借用tensorflow 等框架代码
时间: 2024-09-24 14:20:41 浏览: 44
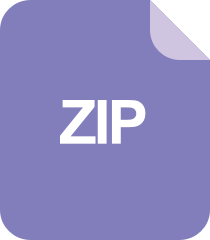
卷积神经网络实现手写数字识别(纯numpy实现)-python卷积神经网络代码.zip
在Python中使用NumPy实现Transformer网络是一个复杂的任务,因为Transformer本身就是基于张量操作的,并且通常会利用像TensorFlow或PyTorch这样的深度学习库提供的矩阵乘法、广播等功能。然而,如果你坚持要用纯NumPy来实现,你需要手动处理这些运算。以下是一个简化版的示例,展示了如何构建基本的注意力机制和简单的多层结构,但这并不是一个完整的Transformer模型。
```python
import numpy as np
def dot_product_attention(q, k, v, mask=None):
d_k = q.shape[-1]
scores = np.matmul(q, k.T) / np.sqrt(d_k)
if mask is not None:
scores += -np.inf * (1 - mask)
attention_weights = np.softmax(scores, axis=-1)
output = np.matmul(attention_weights, v)
return output, attention_weights
class PositionalEncoding:
def __init__(self, max_len=512, d_model=512):
self.pos_encoding = np.zeros((max_len, d_model))
for pos in range(max_len):
for i in range(0, d_model, 2):
self.pos_encoding[pos, i] = np.sin(pos / np.power(10000, (2 * i) / d_model))
self.pos_encoding[pos, i + 1] = np.cos(pos / np.power(10000, (2 * i) / d_model))
# 假设我们有4维输入数据 (batch_size, seq_len, embed_dim, heads)
class MultiHeadAttention:
# ...省略头的数量和其他配置...
def forward(self, queries, keys, values, mask=None):
# ...计算q, k, v... (这里只给出了名字,需要你自己实现)
attention_output, att_wts = dot_product_attention(...)
return attention_output
class EncoderLayer:
def __init__(self, mha, ffn):
self.mha = mha
self.ffn = ffn
def forward(self, x, src_mask):
# 自注意力
attn_output = self.mha(x, x, x, src_mask)
# 添加残差连接和层归一化
x = x + attn_output
x = LayerNorm(x)
# FFN部分
ffn_output = self.ffn(x)
x = x + ffn_output
# 再次添加层归一化
x = LayerNorm(x)
return x
# 类似地,你可以创建DecoderLayer并组合成Encoder和Decoder
# 注意这只是基础框架,实际实现还需要更多的细节如masking、position embeddings等
```
**
阅读全文
相关推荐
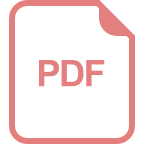
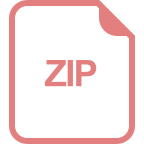















