opencv读取指定文件夹的指定图片
时间: 2024-09-16 08:03:10 浏览: 69
OpenCV(Open Source Computer Vision Library)是一个开源库,用于图像和视频处理。要使用OpenCV读取特定文件夹中的特定图片,你可以按照以下步骤操作:
1. 导入必要的库[^4]:
```python
import cv2
import os
```
2. 定义文件夹路径和图片名称:
```python
folder_path = "/path/to/your/folder" # 替换为实际文件夹路径
image_name = "example.jpg" # 替换为你想要读取的图片名称
```
3. 使用`cv2.imread()`函数读取图片,注意如果图片不在当前工作目录下,需要提供完整的路径[^4]:
```python
# 如果图片位于指定文件夹内
full_image_path = os.path.join(folder_path, image_name)
image = cv2.imread(full_image_path)
# 如果图片路径不正确,可能会抛出异常
if image is not None:
# 图片读取成功,可以继续进行后续处理
else:
print(f"Failed to read the image at {full_image_path}")
```
相关问题
请用C#生成一段代码:通过button控件触发OpenCvSharp读取指定文件夹的第二张图片
在C#中,你可以使用OpenCVSharp库结合Windows Forms中的Button控件来实现这个功能。首先,你需要添加OpenCVSharp NuGet包到项目中。以下是一个简单的示例,展示如何在按钮点击事件中读取指定文件夹的第二张图片:
```csharp
using OpenCvSharp;
using System.IO;
public partial class Form1 : Form
{
private readonly string _imageFolder = "path_to_your_folder"; // 替换为你图片的实际路径
public Form1()
{
InitializeComponent();
LoadImages(); // 初始化图片列表
}
private List<Mat> imagesList; // 存储图片的列表
private void LoadImages()
{
if (!Directory.Exists(_imageFolder))
return;
imagesList = new List<Mat>();
int index = 0;
foreach (string filePath in Directory.GetFiles(_imageFolder))
{
using (var img = Cv2.ImRead(filePath))
{
if (img != null)
imagesList.Add(img);
else
Console.WriteLine($"Failed to load image {filePath}");
// 只读取前两张图片
if (index >= 2)
break;
index++;
}
}
}
private void button1_Click(object sender, EventArgs e)
{
if (imagesList.Count > 1)
{
Mat secondImage = imagesList[1]; // 读取第二张图片
// 在这里可以对图片进行处理,如显示、保存等
// Cv2.imshow("Second Image", secondImage); // 显示图片
// Cv2.waitKey(0); // 等待用户按键
}
else
{
MessageBox.Show("No second image available.");
}
}
}
```
在这个例子中,`button1_Click`方法会在用户点击Button时被调用,如果已经加载了至少两张图片,则读取并显示第二张图片。记得替换`_imageFolder`变量为你实际的图片存储目录。
opencvsharp读取文件夹图片,并将图片的地址保存在一个string[]数组里
在使用OpenCVSharp库读取文件夹中的图片并将它们的路径存储到String数组中,你需要遵循以下步骤:
首先,确保你已经安装了OpenCVSharp库,可以使用NuGet包管理器添加。
1. 引入必要的命名空间:
```csharp
using OpenCvSharp;
using System.IO;
using System.Collections.Generic;
```
2. 定义一个函数来遍历文件夹并收集图片路径:
```csharp
public static string[] GetImagePaths(string folderPath)
{
List<string> imagePaths = new List<string>();
if (!Directory.Exists(folderPath))
{
Console.WriteLine($"The folder path {folderPath} does not exist.");
return imagePaths; // 返回空数组或抛出异常
}
foreach (string filePath in Directory.GetFiles(folderPath, "*.jpg", SearchOption.AllDirectories))
{
// 只考虑.jpg格式的图片,你可以根据需要调整这里
if (System.IO.Path.GetExtension(filePath) == ".jpg")
imagePaths.Add(filePath);
}
return imagePaths.ToArray(); // 转换为数组返回
}
```
在这个函数中,`Directory.GetFiles`用于获取指定文件夹下的所有`.jpg`文件,然后将路径添加到`List<string>`中。最后,我们将其转换回字符串数组返回。
使用这个函数的方式如下:
```csharp
string folderPath = "your_folder_path";
string[] imageFiles = GetImagePaths(folderPath);
foreach (string imagePath in imageFiles)
{
Console.WriteLine(imagePath); // 打印每张图片的路径
}
```
阅读全文
相关推荐
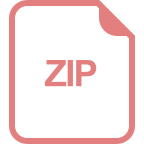
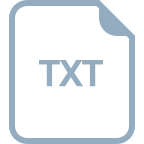
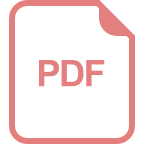
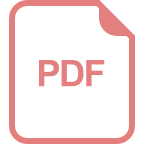
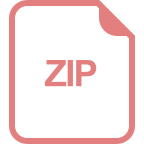
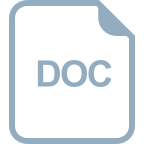









