使用select系统调用的方式接收vblank信号 代码
时间: 2024-03-27 19:41:26 浏览: 12
以下是一个使用 select 系统调用接收 vblank 信号的简单示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/select.h>
#include <sys/ioctl.h>
#include <drm/drm.h>
#include <drm/drm_mode.h>
int main() {
int fd, ret;
fd_set fds;
struct timeval tv;
drmEventContext evctx = {
.version = DRM_EVENT_CONTEXT_VERSION,
.vblank_handler = NULL,
.page_flip_handler = NULL,
};
drmVBlank vbl;
// 打开 DRM 设备文件
fd = open("/dev/dri/card0", O_RDWR);
if (fd < 0) {
perror("open");
exit(EXIT_FAILURE);
}
// 注册 vblank 事件
vbl.request.type = DRM_VBLANK_RELATIVE;
vbl.request.sequence = 1;
ret = ioctl(fd, DRM_IOCTL_WAIT_VBLANK, &vbl);
if (ret < 0) {
perror("ioctl");
exit(EXIT_FAILURE);
}
// 将 vblank 事件转换为文件描述符
ret = drmHandleEvent(fd, &evctx);
if (ret < 0) {
perror("drmHandleEvent");
exit(EXIT_FAILURE);
}
// 将 vblank 事件的文件描述符添加到 select 监视的文件描述符集合中
FD_ZERO(&fds);
FD_SET(fd, &fds);
// 等待 vblank 事件的发生
tv.tv_sec = 5;
tv.tv_usec = 0;
ret = select(fd + 1, &fds, NULL, NULL, &tv);
if (ret < 0) {
perror("select");
exit(EXIT_FAILURE);
}
else if (ret == 0) {
printf("timeout\n");
}
else if (ret > 0) {
if (FD_ISSET(fd, &fds)) {
printf("vblank event received\n");
}
}
// 关闭 DRM 设备文件
close(fd);
return 0;
}
```
需要注意的是,这只是一个简单的示例代码,实际应用程序可能需要更复杂的逻辑来处理 vblank 事件。此外,由于不同的操作系统和显卡驱动可能会有不同的实现方式,因此在实际应用程序中,你可能需要根据具体情况进行调整。
相关推荐
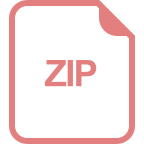
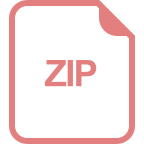














