golang gonum
时间: 2023-10-02 22:05:53 浏览: 112
Gonum是一个用于科学计算的Go语言软件库。它提供了许多常用的数学函数和工具,包括线性代数、概率分布、优化、图形数据结构等。
Gonum的目标是成为Go语言中最全面和最可靠的数学库之一。它使用了现代的编程技术和高效的算法,以提供高性能和可靠的计算功能。
如果您需要在Go语言中进行科学计算或数学建模,Gonum是一个值得考虑的选择。
相关问题
golang gonum resample
Gonum is a numerical library for the Go programming language, and it includes a resampling package for signal processing called `gonum/dsp/resample`. This package provides functions for resampling signals, which involves changing the sample rate of a signal while preserving its frequency content.
To use the `gonum/dsp/resample` package, you first need to create a resampler object by calling the `New` function and passing in the input and output sample rates:
```go
import "gonum.org/v1/gonum/dsp/resample"
inputRate := 44100.0
outputRate := 48000.0
resampler := resample.New(inputRate, outputRate)
```
You can then use the resampler object to resample a signal by calling the `Resample` function and passing in the input and output signals:
```go
input := []float64{...}
output := make([]float64, ...)
resampler.Resample(output, input)
```
The `Resample` function modifies the output slice in place, so you need to make sure it has enough capacity to hold the resampled signal.
There are also other options you can set on the resampler object, such as the resampling algorithm used and the number of filter taps used. You can find more information on these options in the `gonum/dsp/resample` documentation.
golang gonum example
Here is an example of using the Gonum library in Go to perform matrix operations:
```go
package main
import (
"fmt"
"gonum.org/v1/gonum/mat"
)
func main() {
// Create a 3x3 matrix
data := []float64{1, 2, 3, 4, 5, 6, 7, 8, 9}
matrix := mat.NewDense(3, 3, data)
// Print the matrix
fmt.Println("Original Matrix:")
matPrint(matrix)
// Multiply the matrix by 2
matrix.Scale(2, matrix)
// Print the result
fmt.Println("Multiplied Matrix:")
matPrint(matrix)
// Get the transpose of the matrix
transpose := mat.NewDense(0, 0, nil)
transpose.TCopy(transpose, matrix)
// Print the result
fmt.Println("Transposed Matrix:")
matPrint(transpose)
// Calculate the determinant of the matrix
determinant := mat.Det(matrix)
// Print the result
fmt.Printf("Determinant: %.2f\n", determinant)
}
// matPrint prints a matrix to stdout.
func matPrint(m mat.Matrix) {
f := mat.Formatted(m, mat.Prefix(""), mat.Squeeze())
fmt.Printf("%v\n", f)
}
```
This program creates a 3x3 matrix and performs several operations on it using the Gonum library. It multiplies the matrix by 2, gets the transpose of the matrix, calculates the determinant of the matrix, and prints the results to the console.
阅读全文
相关推荐
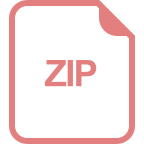
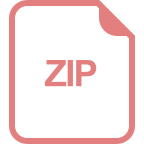
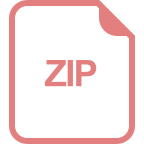
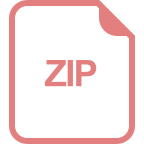
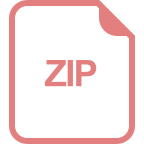
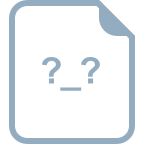
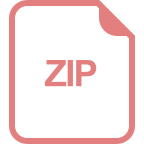
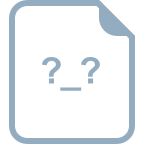






