用python实现 将输入的骨架化的图像进行曲线拟合 批量图片输入和批量图片保存
时间: 2024-05-15 22:19:42 浏览: 107
实现思路:
1. 读取输入的骨架化图像;
2. 对图像进行二值化处理;
3. 用OpenCV中的findContours函数找到图像中的轮廓;
4. 对每个轮廓进行曲线拟合;
5. 将拟合后的曲线绘制在一张空白图像上;
6. 将拟合后的曲线绘制在原图像上,形成拟合后的图像;
7. 将拟合后的图像保存。
代码实现:
```python
import cv2
import numpy as np
import os
def curve_fitting(image_path, save_path):
# 读取输入的骨架化图像
img = cv2.imread(image_path, 0)
# 对图像进行二值化处理
ret, thresh = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY)
# 用findContours函数找到图像中的轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 创建一张空白图像
blank_img = np.zeros((img.shape[0], img.shape[1], 3), np.uint8)
# 对每个轮廓进行曲线拟合
for i in range(len(contours)):
cnt = contours[i]
if len(cnt) > 10:
# 对轮廓进行多项式拟合,拟合出3次曲线
x, y = cnt[:, 0, 0], cnt[:, 0, 1]
z = np.polyfit(x, y, 3)
p = np.poly1d(z)
x_new = np.linspace(x[0], x[-1], 100)
y_new = p(x_new)
# 将拟合后的曲线绘制在一张空白图像上
for j in range(len(x_new)):
cv2.circle(blank_img, (int(x_new[j]), int(y_new[j])), 1, (255, 255, 255), -1)
# 将拟合后的曲线绘制在原图像上,形成拟合后的图像
cv2.polylines(img, [np.int32(np.column_stack((x_new, y_new)))], False, (255, 255, 255), 1)
# 将拟合后的图像保存
cv2.imwrite(save_path, img)
if __name__ == '__main__':
# 批量图片输入和批量图片保存
input_folder = './input_folder/'
output_folder = './output_folder/'
for image_name in os.listdir(input_folder):
image_path = input_folder + image_name
save_path = output_folder + image_name
curve_fitting(image_path, save_path)
```
使用说明:
1. 将需要处理的骨架化图像放在input_folder文件夹下;
2. 运行上述代码,即可得到拟合后的图像,并保存在output_folder文件夹下。
阅读全文
相关推荐
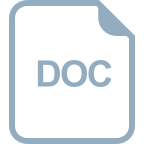
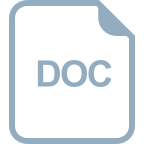
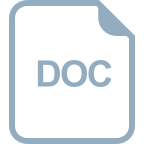


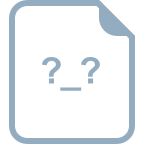
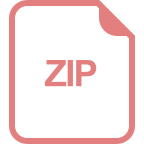
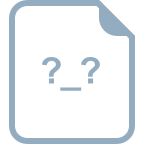
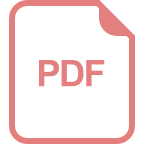
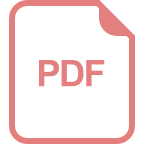
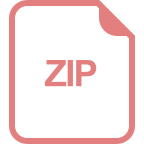
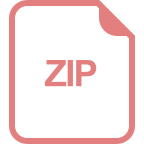
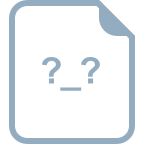
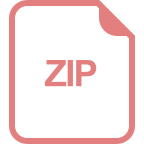
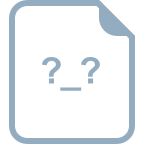
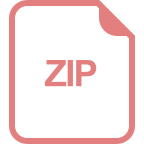
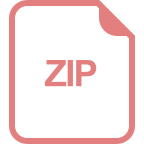
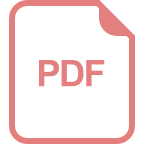