用python实现 将输入的骨架化的图像进行曲线拟合 批量图片输入和批量图片保
时间: 2024-04-30 17:22:18 浏览: 223
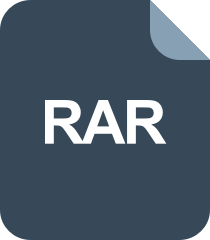
Python图片批量处理器
存
实现步骤:
1. 导入必要的库,包括opencv、numpy、os等
2. 定义函数,读取文件夹中的所有图片并进行骨架化处理
3. 定义函数,将骨架化图像进行曲线拟合,并输出拟合后的图像
4. 定义函数,将拟合后的图像保存到指定文件夹中
5. 调用函数,实现批量处理图片
代码如下:
```python
import cv2
import numpy as np
import os
# 读取文件夹中的所有图片并进行骨架化处理
def skel_batch(folder_path):
# 获取文件夹中的所有图片路径
file_list = os.listdir(folder_path)
for file in file_list:
# 判断是否为图片文件
if file.endswith('.jpg') or file.endswith('.png'):
# 读取图片
img = cv2.imread(os.path.join(folder_path, file))
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 骨架化
size = np.size(thresh)
skel = np.zeros(thresh.shape, np.uint8)
element = cv2.getStructuringElement(cv2.MORPH_CROSS, (3, 3))
done = False
while not done:
eroded = cv2.erode(thresh, element)
temp = cv2.dilate(eroded, element)
temp = cv2.subtract(thresh, temp)
skel = cv2.bitwise_or(skel, temp)
thresh = eroded.copy()
zeros = size - cv2.countNonZero(thresh)
if zeros == size:
done = True
# 显示骨架化图像
cv2.imshow('Skeleton', skel)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 将骨架化图像进行曲线拟合,并输出拟合后的图像
def curve_fitting(skel):
# 轮廓检测
contours, hierarchy = cv2.findContours(skel, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)
# 提取轮廓
cnt = contours[0]
# 进行曲线拟合
rows, cols = skel.shape
[vx, vy, x, y] = cv2.fitLine(cnt, cv2.DIST_L2, 0, 0.01, 0.01)
lefty = int((-x*vy/vx) + y)
righty = int(((cols-x)*vy/vx)+y)
# 绘制直线
line = cv2.line(skel,(cols-1,righty),(0,lefty),(255,255,255),1)
# 显示拟合后的图像
cv2.imshow('Fitting', line)
cv2.waitKey(0)
cv2.destroyAllWindows()
return line
# 将拟合后的图像保存到指定文件夹中
def save_batch(folder_path, save_path):
# 获取文件夹中的所有图片路径
file_list = os.listdir(folder_path)
for file in file_list:
# 判断是否为图片文件
if file.endswith('.jpg') or file.endswith('.png'):
# 读取图片
img = cv2.imread(os.path.join(folder_path, file))
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 骨架化
size = np.size(thresh)
skel = np.zeros(thresh.shape, np.uint8)
element = cv2.getStructuringElement(cv2.MORPH_CROSS, (3, 3))
done = False
while not done:
eroded = cv2.erode(thresh, element)
temp = cv2.dilate(eroded, element)
temp = cv2.subtract(thresh, temp)
skel = cv2.bitwise_or(skel, temp)
thresh = eroded.copy()
zeros = size - cv2.countNonZero(thresh)
if zeros == size:
done = True
# 进行曲线拟合
line = curve_fitting(skel)
# 保存拟合后的图像
cv2.imwrite(os.path.join(save_path, file), line)
# 批量处理图片
folder_path = './images' # 输入文件夹
save_path = './result' # 输出文件夹
skel_batch(folder_path) # 骨架化处理
save_batch(folder_path, save_path) # 曲线拟合并输出
```
注意事项:
1. 需要提前创建好输入文件夹和输出文件夹
2. 输入文件夹中的图片必须为jpg或png格式的
3. 输出文件夹中的图片格式与输入文件夹中的图片格式相同
阅读全文
相关推荐
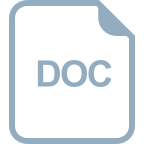
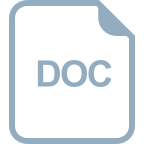



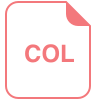
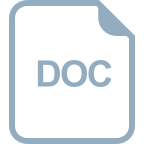
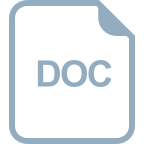
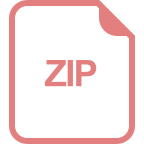
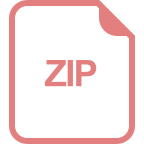
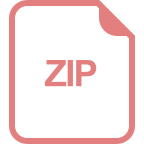
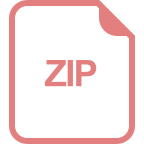