public partial class Form1 : Form { private ushort _CardID = 0; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { short num = LTDMC.dmc_board_init();//获取卡数量 if (num <= 0 || num > 8) { MessageBox.Show("初始卡失败!", "出错"); } ushort _num = 0; ushort[] cardids = new ushort[8]; uint[] cardtypes = new uint[8]; short res = LTDMC.dmc_get_CardInfList(ref _num, cardtypes, cardids); if (res != 0) { MessageBox.Show("获取卡信息失败!"); } _CardID = cardids[0]; // timer1.Start(); }
时间: 2024-02-10 11:22:40 浏览: 233
这段代码是一个 Windows 窗体应用程序中的 Form1 类,它的 Load 事件处理程序。该处理程序使用了 LTDMC 库来初始化运动控制卡,并获取卡的信息。
首先,使用 LTDMC 库中的 dmc_board_init() 函数获取卡数量,如果数量不在 1 到 8 之间,则显示一个错误消息框。
然后,使用 LTDMC 库中的 dmc_get_CardInfList() 函数获取每个卡的类型和 ID,如果获取失败,则显示一个错误消息框。
最后,将第一个卡的 ID 存储在私有变量 _CardID 中,并启动一个计时器 timer1。
这段代码的目的是初始化运动控制卡,并获取第一个卡的 ID,以便在后续的代码中使用。
相关问题
讲代码补充完整using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; namespace one { public partial class Form1 : Form { private Classes mycla = new Classes(); public Form1() { InitializeComponent(); } private void List() { // Student s; textBox3.Text = ""; for (int i = 1; i <=mycla.Count; i++) { //编写代码 //.... // textBox3.Text += s.code + "\t"+s.Name+"\r\n"; } } private void button1_Click(object sender, EventArgs e) { //编写程序 List();//显示学生信息 } private void button2_Click(object sender, EventArgs e) { //编写代码 } } }
以上是一个Windows Forms应用程序的部分代码,包含一个窗体类Form1和一个Classes类对象mycla。下面是将其使用的代码补充完整:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace one
{
public partial class Form1 : Form
{
private Classes mycla = new Classes();
public Form1()
{
InitializeComponent();
}
private void List()
{
textBox3.Text = "";
for (int i = 1; i <= mycla.Count; i++)
{
Student s = mycla.GetStudent(i);
textBox3.Text += s.code + "\t" + s.Name + "\r\n";
}
}
private void button1_Click(object sender, EventArgs e)
{
List();
}
private void button2_Click(object sender, EventArgs e)
{
string code = textBox1.Text;
string name = textBox2.Text;
Student s = new Student(code, name);
mycla.AddStudent(s);
textBox1.Text = "";
textBox2.Text = "";
List();
}
}
}
在List方法中,我们使用for循环遍历mycla对象中所有的学生信息,并且将每个学生的学号和姓名信息打印在textBox3中。
在button2_Click方法中,我们通过textBox1和textBox2获取用户输入的学号和姓名信息,并且创建一个新的学生对象s,将其添加到mycla对象中。最后清空textBox1和textBox2,并且调用List方法刷新显示。
public partial class Form1 : Form{ private Point mouseOffset; // 鼠标位置与图片位置的差值 private Point imageLocation; // 图片的位置 public Form1() { InitializeComponent(); } private void pictureBox1_MouseDown(object sender, MouseEventArgs e) { if (e.Button == MouseButtons.Left) { mouseOffset = new Point(-e.X, -e.Y); imageLocation = pictureBox1.ImageLocation; } } private void pictureBox1_MouseMove(object sender, MouseEventArgs e) { if (e.Button == MouseButtons.Left) { Point mousePos = Control.MousePosition; mousePos.Offset(mouseOffset.X, mouseOffset.Y); pictureBox1.ImageLocation = imageLocation; pictureBox1.ImageLocation = mousePos; } } private void pictureBox1_MouseUp(object sender, MouseEventArgs e) { mouseOffset = Point.Empty; imageLocation = Point.Empty; }}报错了
这段代码的报错信息可能是因为 `pictureBox1` 控件的 `ImageLocation` 属性为只读属性,不能直接对其赋值。你可以尝试修改为 `pictureBox1.Location` 属性来实现鼠标拖动图片的效果。
修改后的代码如下:
```
public partial class Form1 : Form
{
private Point mouseOffset; // 鼠标位置与图片位置的差值
private Point imageLocation; // 图片的位置
public Form1()
{
InitializeComponent();
}
private void pictureBox1_MouseDown(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
mouseOffset = new Point(-e.X, -e.Y);
imageLocation = pictureBox1.Location;
}
}
private void pictureBox1_MouseMove(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
Point mousePos = Control.MousePosition;
mousePos.Offset(mouseOffset.X, mouseOffset.Y);
pictureBox1.Location = mousePos;
}
}
private void pictureBox1_MouseUp(object sender, MouseEventArgs e)
{
mouseOffset = Point.Empty;
imageLocation = Point.Empty;
}
}
```
希望这能帮助到你!
阅读全文
相关推荐
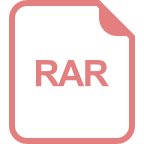
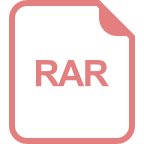
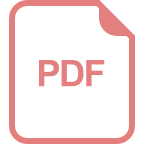













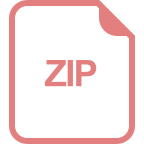