namespace WinFormsApp1 { public partial class Form1 : Form //定义一个公共的部分类Form1,Form1继承自另一个类Form { CCircle cir; CCylinder cyl; CCone con; public Form1() { InitializeComponent(); cir = new CCircle() { r = 1 }; cyl = new CCylinder() { c = cir, h = 1 }; con = new CCone() { c = cir, h = 1 }; } private void button1_Click(object sender, EventArgs e) { cir.r = (double)numericUpDown1.Value; double a = cir.GetArea(); MessageBox.Show("圆面积" + a); } private void button2_Click(object sender, EventArgs e) { cyl.h = (double)numericUpDown2.Value; double v = cyl.GetVolume(); MessageBox.Show("圆柱体体积" + v); } private void button3_Click(object sender, EventArgs e) { con.h = (double)numericUpDown2.Value; double v = con.GetVolume(); MessageBox.Show("圆锥体体积" + v); } }
时间: 2023-05-18 14:03:40 浏览: 229
这段代码定义了一个名为 Form1 的窗体类,它包含了三个对象:CCircle、CCylinder 和 CCone。在窗体的构造函数中,这三个对象都被初始化了。在窗体中,有三个按钮,分别对应着计算圆的面积、圆柱体的体积和圆锥体的体积。当用户点击这些按钮时,相应的对象的属性会被更新,并计算出相应的面积或体积,然后在消息框中显示出来。
相关问题
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; //添加ABB库引用 using ABB.Robotics.Controllers; using ABB.Robotics.Controllers.Discovery; using ABB.Robotics; namespace RobotManager2 { public partial class Form1 : Form { //扫描器 private NetworkScanner scanner=null; //控制器集合 private ControllerInfoCollection controllers=null; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { } void ScanConroller() { //创建扫描器 this.scanner = new NetworkScanner(); //启动扫描 this.scanner.Scan(); } } }
这段代码是一个 C# 的 Windows Forms 应用程序,其中使用了 ABB 公司的机器人控制器库。主要实现了以下功能:
1. 创建了一个窗体 Form1;
2. 实现了窗体加载事件 Form1_Load;
3. 创建了一个 NetworkScanner 对象 scanner,用于扫描网络中可用的机器人控制器;
4. 创建了一个 ControllerInfoCollection 对象 controllers,用于存储扫描到的控制器信息。
在 Form1_Load 事件中,可以调用 ScanConroller 方法来启动扫描器,获取可用的机器人控制器列表。此处只是代码的一部分,如果需要使用 ABB 机器人控制器库进行开发,还需要补充其他代码来实现具体的功能。
using System.IO.Ports; namespace Pan_Port { public partial class Form1 : Form { private SerialPort serialPort; 发送 fe 08 00 0a 00 00 00 00
你可以使用 `serialPort` 对象来发送数据。根据你提供的代码,我假设你已经在 `Form1` 类中正确地初始化了 `serialPort` 对象。下面是一个示例,演示如何使用 `serialPort` 对象发送数据:
```csharp
using System.IO.Ports;
namespace Pan_Port
{
public partial class Form1 : Form
{
private SerialPort serialPort;
public Form1()
{
InitializeComponent();
InitializeSerialPort();
SendData();
}
private void InitializeSerialPort()
{
serialPort = new SerialPort();
serialPort.PortName = "COM1"; // 设置串口号
serialPort.BaudRate = 9600; // 设置波特率
serialPort.DataBits = 8; // 设置数据位
serialPort.StopBits = StopBits.One; // 设置停止位
serialPort.Parity = Parity.None; // 设置校验位
try
{
serialPort.Open(); // 打开串口连接
// 其他串口初始化操作...
}
catch (Exception ex)
{
// 处理串口打开异常...
MessageBox.Show("串口打开失败: " + ex.Message);
}
}
private void SendData()
{
byte[] data = new byte[] { 0xfe, 0x08, 0x00, 0x0a, 0x00, 0x00, 0x00, 0x00 };
try
{
serialPort.Write(data, 0, data.Length); // 发送数据
// 其他处理发送成功的操作...
}
catch (Exception ex)
{
// 处理发送失败的异常...
MessageBox.Show("发送数据失败: " + ex.Message);
}
}
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
if (serialPort != null && serialPort.IsOpen)
{
serialPort.Close(); // 关闭串口连接
}
}
base.Dispose(disposing);
}
}
}
```
在上述示例中,我们添加了一个名为 `SendData` 的方法,用于发送数据。我们创建了一个 `byte` 数组 `data`,其中包含要发送的数据。然后,我们使用 `serialPort.Write` 方法将数据发送到串口。请注意,这只是一个示例,你可以根据实际需求修改和扩展该方法。
希望能解答你的问题!如果还有其他疑问,请随时提问。
阅读全文
相关推荐
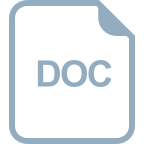
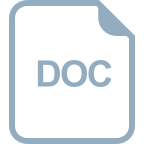
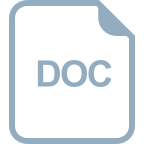














