使用线程模拟餐厅的运营(必须用线程池): 服务员: 1. 餐厅有两位长期服务员 (核心线程) 2. 餐厅可以多招一个临时服务员 3. 临时服务员如果六十秒没活干就炒掉 4. 在餐厅门外只有十个位置让客人等待, 满了再来客直接抛异常 AbortPolicy 流程: 1. 每位服务员都需要去厨房取食物 2. 厨房需要预备食物让服务员领取 - 只有一位厨师预备食物 - 每秒随机炒一个菜, 最多可存十个菜 3. 每次只能一位服务员进厨房, 取得食物后打印出食物名称, 耗时三秒 进阶: 用Scanner 提供用户介面 1. 食客命令开始上菜, 菜品直接从厨房拿随机的, 不断运行 2. 食客手动不断点菜, 直至他想自己离开,请用java代码实现
时间: 2023-03-25 21:00:44 浏览: 163
以下是使用线程池模拟餐厅运营的Java代码:
import java.util.concurrent.*;
public class RestaurantSimulation {
public static void main(String[] args) {
// 创建线程池
ExecutorService executor = Executors.newFixedThreadPool(3);
// 创建餐厅对象
Restaurant restaurant = new Restaurant();
// 创建服务员对象
Waiter waiter1 = new Waiter(restaurant);
Waiter waiter2 = new Waiter(restaurant);
Waiter tempWaiter = new Waiter(restaurant);
tempWaiter.setTemporary(true);
// 提交服务员任务到线程池
executor.submit(waiter1);
executor.submit(waiter2);
executor.submit(tempWaiter);
// 创建厨师对象
Chef chef = new Chef(restaurant);
// 提交厨师任务到线程池
executor.submit(chef);
// 创建用户界面对象
UserInterface ui = new UserInterface(restaurant);
// 提交用户界面任务到线程池
executor.submit(ui);
// 关闭线程池
executor.shutdown();
}
}
// 餐厅类
class Restaurant {
private BlockingQueue<String> foodQueue; // 食物队列
private int waitingListSize; // 等待队列大小
private Semaphore kitchenSemaphore; // 厨房信号量
public Restaurant() {
foodQueue = new ArrayBlockingQueue<>(10);
waitingListSize = 10;
kitchenSemaphore = new Semaphore(1);
}
public BlockingQueue<String> getFoodQueue() {
return foodQueue;
}
public int getWaitingListSize() {
return waitingListSize;
}
public Semaphore getKitchenSemaphore() {
return kitchenSemaphore;
}
}
// 服务员类
class Waiter implements Runnable {
private Restaurant restaurant;
private boolean isTemporary;
public Waiter(Restaurant restaurant) {
this.restaurant = restaurant;
this.isTemporary = false;
}
public void setTemporary(boolean temporary) {
isTemporary = temporary;
}
@Override
public void run() {
while (true) {
try {
// 从等待队列中取出一个客人
String customer = restaurant.getFoodQueue().take();
// 进入厨房
restaurant.getKitchenSemaphore().acquire();
// 取出一份食物
String food = restaurant.getFoodQueue().take();
// 打印食物名称
System.out.println(Thread.currentThread().getName() + " 上菜:" + food);
// 离开厨房
restaurant.getKitchenSemaphore().release();
// 如果是临时服务员,等待60秒后退出
if (isTemporary) {
Thread.sleep(60000);
break;
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
// 厨师类
class Chef implements Runnable {
private Restaurant restaurant;
public Chef(Restaurant restaurant) {
this.restaurant = restaurant;
}
@Override
public void run() {
while (true) {
try {
// 随机炒一个菜
String food = "菜品" + (int) (Math.random() * 100);
// 进入厨房
restaurant.getKitchenSemaphore().acquire();
// 如果食物队列已满,等待
while (restaurant.getFoodQueue().remainingCapacity() == ) {
Thread.sleep(100);
}
// 将食物放入队列
restaurant.getFoodQueue().put(food);
// 离开厨房
restaurant.getKitchenSemaphore().release();
// 等待一秒
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
// 用户界面类
class UserInterface implements Runnable {
private Restaurant restaurant;
public UserInterface(Restaurant restaurant) {
this.restaurant = restaurant;
}
@Override
public void run() {
while (true) {
try {
// 从控制台读取用户输入
String input = new java.util.Scanner(System.in).nextLine();
// 如果用户输入了"exit",退出程序
if (input.equals("exit")) {
System.exit();
}
// 如果用户输入了"start",开始上菜
if (input.equals("start")) {
while (true) {
// 如果等待队列已满,抛出异常
if (restaurant.getFoodQueue().remainingCapacity() == ) {
throw new RejectedExecutionException("等待队列已满");
}
// 将客人加入等待队列
restaurant.getFoodQueue().put("客人");
// 等待一秒
Thread.sleep(100);
}
}
// 如果用户输入了其他内容,点菜
String food = "菜品" + (int) (Math.random() * 100);
System.out.println("点菜:" + food);
restaurant.getFoodQueue().put(food);
} catch (InterruptedException e) {
e.printStackTrace();
} catch (RejectedExecutionException e) {
System.out.println(e.getMessage());
}
}
}
}
阅读全文
相关推荐

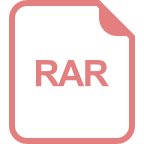











