c语言代码游戏五子棋
时间: 2023-08-09 16:05:24 浏览: 48
好的,下面是一个简单的C语言代码实现的五子棋游戏。在这个游戏中,玩家和电脑轮流下棋,先连成五子的一方获胜。以下是完整的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define SIZE 15
int board[SIZE][SIZE]; // 棋盘
int player = 1; // 玩家执黑子,电脑执白子
// 初始化棋盘
void init_board()
{
int i, j;
for (i = 0; i < SIZE; i++)
{
for (j = 0; j < SIZE; j++)
{
board[i][j] = 0;
}
}
}
// 显示棋盘
void display_board()
{
int i, j;
printf(" ");
for (i = 0; i < SIZE; i++)
{
printf(" %d", i);
}
printf("\n");
for (i = 0; i < SIZE; i++)
{
printf("%2d", i);
for (j = 0; j < SIZE; j++)
{
if (board[i][j] == 0)
{
printf(" .");
}
else if (board[i][j] == 1)
{
printf(" X");
}
else
{
printf(" O");
}
}
printf("\n");
}
}
// 判断是否有五子连珠
int check_win(int x, int y)
{
int i, j, count;
// 横向判断
count = 0;
for (i = y - 4; i <= y; i++)
{
if (i < 0 || i + 4 >= SIZE) continue;
count = 0;
for (j = i; j <= i + 4; j++)
{
if (board[x][j] == player)
{
count++;
}
}
if (count == 5) return 1;
}
// 纵向判断
count = 0;
for (i = x - 4; i <= x; i++)
{
if (i < 0 || i + 4 >= SIZE) continue;
count = 0;
for (j = i; j <= i + 4; j++)
{
if (board[j][y] == player)
{
count++;
}
}
if (count == 5) return 1;
}
// 左上到右下判断
count = 0;
for (i = x - 4, j = y - 4; i <= x && j <= y; i++, j++)
{
if (i < 0 || j < 0 || i + 4 >= SIZE || j + 4 >= SIZE) continue;
count = 0;
for (int k = 0; k < 5; k++)
{
if (board[i+k][j+k] == player)
{
count++;
}
}
if (count == 5) return 1;
}
// 左下到右上判断
count = 0;
for (i = x + 4, j = y - 4; i >= x && j <= y; i--, j++)
{
if (i >= SIZE || j < 0 || i - 4 < 0 || j + 4 >= SIZE) continue;
count = 0;
for (int k = 0; k < 5; k++)
{
if (board[i-k][j+k] == player)
{
count++;
}
}
if (count == 5) return 1;
}
return 0;
}
// 玩家下棋
void player_move()
{
int x, y;
printf("请输入你要落子的位置(x y):");
scanf("%d %d", &x, &y);
while (board[x][y] != 0)
{
printf("这个位置已经有棋子了,请重新输入(x y):");
scanf("%d %d", &x, &y);
}
board[x][y] = 1;
}
// 电脑下棋
void computer_move()
{
int x, y;
srand(time(0));
do
{
x = rand() % SIZE;
y = rand() % SIZE;
} while (board[x][y] != 0);
printf("电脑在(%d, %d)处落子\n", x, y);
board[x][y] = 2;
}
int main()
{
int round = 0;
init_board();
display_board();
while (1)
{
if (player == 1)
{
player_move();
if (check_win(x, y))
{
printf("你赢了!\n");
break;
}
player = 2;
}
else
{
computer_move();
if (check_win(x, y))
{
printf("电脑赢了!\n");
break;
}
player = 1;
}
round++;
display_board();
if (round == SIZE * SIZE)
{
printf("平局!\n");
break;
}
}
return 0;
}
```
在这个游戏中,我们使用一个二维数组来表示棋盘,其中1代表黑子,2代表白子,0代表空位。玩家和电脑轮流下棋,每次下棋后判断是否有五子连珠,如果有,则获胜。如果棋盘上没有空位了,判定为平局。
相关推荐
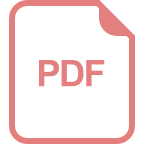














