C语言简单版五子棋悔棋代码
时间: 2023-09-04 14:07:14 浏览: 129
以下是一个简单的 C 语言五子棋悔棋代码示例,使用了栈的数据结构来实现:
c
#include <stdio.h#include <stdlib.h>
#define ROW 15
#define COL 15
#define CHESS_NUM 5
typedef struct {
int x;
int y;
} Chess;
typedef struct {
Chess data[ROW * COL];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -;
}
int isFull(Stack *s) {
return s->top == ROW * COL - 1;
}
void push(Stack *s, Chess c) {
if (isFull(s)) {
printf("Stack is full!\n");
exit(0);
}
s->data[++s->top] = c;
}
Chess pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty!\n");
exit(0);
}
return s->data[s->top--];
}
void printBoard(char board[ROW][COL]) {
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
printf("%c ", board[i][j]);
}
printf("\n");
}
}
int checkWin(char board[ROW][COL], Chess c) {
int i, j, k;
int count;
// 横向检查
for (i = c.y - CHESS_NUM + 1; i <= c.y; i++) {
if (i < 0 || i + CHESS_NUM > COL) {
continue;
}
count = 0;
for (j = i; j < i + CHESS_NUM; j++) {
if (board[c.x][j] == board[c.x][c.y]) {
count++;
}
}
if (count == CHESS_NUM) {
return 1;
}
}
// 纵向检查
for (i = c.x - CHESS_NUM + 1; i <= c.x; i++) {
if (i < 0 || i + CHESS_NUM > ROW) {
continue;
}
count = 0;
for (j = i; j < i + CHESS_NUM; j++) {
if (board[j][c.y] == board[c.x][c.y]) {
count++;
}
}
if (count == CHESS_NUM) {
return 1;
}
}
// 左上 - 右下检查
for (i = c.x - CHESS_NUM + 1, j = c.y - CHESS_NUM + 1; i <= c.x && j <= c.y; i++, j++) {
if (i < 0 || j < 0 || i + CHESS_NUM > ROW || j + CHESS_NUM > COL) {
continue;
}
count = 0;
for (k = 0; k < CHESS_NUM; k++) {
if (board[i + k][j + k] == board[c.x][c.y]) {
count++;
}
}
if (count == CHESS_NUM) {
return 1;
}
}
// 右上 - 左下检查
for (i = c.x - CHESS_NUM + 1, j = c.y + CHESS_NUM - 1; i <= c.x && j >= c.y; i++, j--) {
if (i < 0 || j >= COL || i + CHESS_NUM > ROW || j - CHESS_NUM + 1 < 0) {
continue;
}
count = 0;
for (k = 0; k < CHESS_NUM; k++) {
if (board[i + k][j - k] == board[c.x][c.y]) {
count++;
}
}
if (count == CHESS_NUM) {
return 1;
}
}
return 0;
}
int main() {
char board[ROW][COL] = {0};
Stack s;
Chess c;
int i, j;
init(&s);
while (1) {
// 打印棋盘
printBoard(board);
// 输入棋子坐标
printf("Please enter your move (x y): ");
scanf("%d %d", &c.x, &c.y);
// 检查该位置是否可下棋
if (board[c.x][c.y] != 0) {
printf("This place has already been taken! Please re-enter.\n");
continue;
}
// 下棋,并将该棋子压入栈中
board[c.x][c.y] = 'X';
push(&s, c);
// 检查是否胜利
if (checkWin(board, c)) {
printf("You win!\n");
break;
}
// 悔棋操作
if (!isEmpty(&s)) {
printf("Do you want to regret? (y/n): ");
char answer;
scanf(" %c", &answer);
if (answer == 'y' || answer == 'Y') {
c = pop(&s);
board[c.x][c.y] = 0;
} else {
continue;
}
}
}
return 0;
}
```
该代码实现了一个简单的五子棋游戏,可以在游戏过程中进行悔棋操作。其核心思想是使用栈来保存每一步的棋子坐标,如果需要悔棋,则弹出栈顶元素并在棋盘上将该棋子标记为 0。同时,代码中还实现了一个简单的胜利判断函数,用于检查某个棋子是否连成了五子。
阅读全文
相关推荐
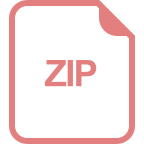
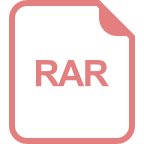
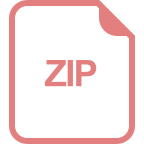















